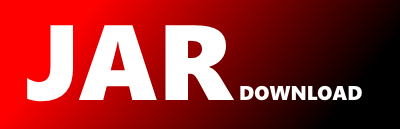
com.gradle.develocity.agent.maven.api.scan.BuildScanDataObfuscation Maven / Gradle / Ivy
Show all versions of develocity-maven-extension Show documentation
package com.gradle.develocity.agent.maven.api.scan;
import java.net.InetAddress;
import java.util.List;
import java.util.function.Function;
/**
* Allows registering functions for obfuscating certain identifying information within build scans.
*
* @see BuildScanApi#getObfuscation()
*/
public interface BuildScanDataObfuscation {
/**
* Registers a function to transform the username captured.
*
* The function receives the username that would be captured,
* and should return the username that will be captured.
*
* The received username may be null
,
* and the function may return a null
value.
*
* @param obfuscator the function to obfuscate the username
*/
void username(Function super String, ? extends String> obfuscator);
/**
* Registers a function to transform the local hostname and public hostname captured.
*
* The function receives the hostname that would be captured,
* and should return the hostname that will be captured.
*
* The received hostname may be null
,
* and the function may return a null
value.
*
* @param obfuscator the function to obfuscate the hostname
*/
void hostname(Function super String, ? extends String> obfuscator);
/**
* Registers a function used to transform the IP addresses captured.
*
* The function receives a list of {@link InetAddress} that would be captured,
* and should return the list of {@link String} that will be captured.
*
* The received list may be null
or empty,
* and the function may return a null
or empty list,
* but may not return a list with a null
element.
*
* @param obfuscator the function to obfuscate the IP addresses
*/
void ipAddresses(Function super List, ? extends List> obfuscator);
/**
* Registers a function to transform the names of external processes captured when resource usage capturing is enabled.
*
* An external process is a process running on the same machine as the build, that is not the build process itself, nor a descendant process of the build process.
* The function receives an external process name that would be captured,
* and should return the external process name that will be captured.
*
* The received process name may not be null
,
* and the function may not return a null
value.
*
* @param obfuscator the function to obfuscate the external process names
* @since 1.22
*/
void externalProcessName(Function super String, ? extends String> obfuscator);
}