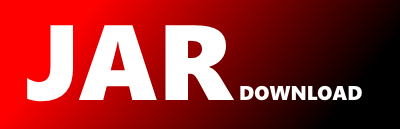
com.gradle.maven.extension.api.cache.NormalizationProvider Maven / Gradle / Ivy
Show all versions of develocity-maven-extension Show documentation
package com.gradle.maven.extension.api.cache;
import org.apache.maven.execution.MavenSession;
import org.apache.maven.project.MavenProject;
import java.util.List;
import java.util.function.Consumer;
/**
* Allows users to configure normalization.
*
* @since 1.15
* @deprecated since 1.21, replaced by {@link com.gradle.develocity.agent.maven.api.cache.NormalizationProvider}
*/
@Deprecated
public interface NormalizationProvider {
/**
* Configure normalization for the project in the supplied context.
*
* This method is called once for each project.
*
* @param context provides access to the project and allows users to customize its runtime classpath normalization
* @since 1.15
*/
void configureNormalization(Context context);
/**
* Allows configuring the normalization of a project.
*
* @since 1.15
*/
interface Context {
/**
* The project being configured.
*
* @since 1.15
*/
MavenProject getProject();
/**
* The session associated with the project.
*
* @since 1.15
*/
MavenSession getSession();
/**
* Allows configuring the runtime classpath normalization.
*
* @param action an action to configure the runtime classpath normalization
* @since 1.15
*/
Context configureRuntimeClasspathNormalization(Consumer action);
/**
* Allows configuring the system property normalization.
*
* @param action an action to configure the system property normalization
* @since 1.18
*/
Context configureSystemPropertiesNormalization(Consumer action);
}
/**
* Allows configuring the runtime classpath normalization.
*
* Supports ANT-style patterns:
*
* * matches zero or more characters
* ? matches one character
* ** matches zero or more characters across directory levels
*
*
* @since 1.15
*/
interface RuntimeClasspathNormalization {
/**
* Configures the runtime classpath normalization to ignore files. Supports ANT-style file paths.
* This operation will override any previous configuration.
*
* @param ignoredFiles the files to ignore
* @since 1.15
*/
RuntimeClasspathNormalization setIgnoredFiles(List ignoredFiles);
/**
* Configures the runtime classpath normalization to ignore files. Supports ANT-style file paths.
* This operation will override any previous configuration.
*
* @param ignoredFiles the files to ignore
* @since 1.15
*/
RuntimeClasspathNormalization setIgnoredFiles(String... ignoredFiles);
/**
* Configures the runtime classpath normalization to ignore files. Supports ANT-style file paths.
* This operation is additive, i.e. it will add the ignored files to the previously configured files
* including the corresponding configuration in POM.
*
* @param ignoredFiles the files to ignore
* @since 1.15
*/
RuntimeClasspathNormalization addIgnoredFiles(List ignoredFiles);
/**
* Configures the runtime classpath normalization to ignore files. Supports ANT-style file paths.
* This operation is additive, i.e. it will add the ignored files to the previously configured files.
*
* @param ignoredFiles the files to ignore
* @since 1.15
*/
RuntimeClasspathNormalization addIgnoredFiles(String... ignoredFiles);
/**
* Configures the runtime classpath normalization to ignore properties from specific property files.
* ANT-style patterns can be used to match relevant property files.
*
* @param path the path of the properties file
* @param ignoredProperties the properties to ignore
* @since 1.15
*/
RuntimeClasspathNormalization addPropertiesNormalization(String path, List ignoredProperties);
/**
* Configures the runtime classpath normalization to ignore properties from specific property files.
* ANT-style patterns can be used to match relevant property files.
*
* @param path the path of the properties file
* @param ignoredProperties the properties to ignore
* @since 1.15
*/
RuntimeClasspathNormalization addPropertiesNormalization(String path, String... ignoredProperties);
/**
* Allows configuring the normalization of files matching META-INF/**/*.properties
.
*
* @param action an action to configure the normalization
* @since 1.15
*/
RuntimeClasspathNormalization configureMetaInf(Consumer action);
/**
* A common location to store properties files is the {@code META-INF} directory.
* Thus, this provides a shortcut for normalizing files matching META-INF/**/*.properties
.
*
* @since 1.15
*/
interface MetaInf {
/**
* Allows ignoring one or more attributes in {@code MANIFEST} files. This operation will override any previous configuration.
*
* @param ignoredAttributes the attributes to ignore
* @since 1.15
*/
RuntimeClasspathNormalization.MetaInf setIgnoredAttributes(List ignoredAttributes);
/**
* Allows ignoring one or more attributes in {@code MANIFEST} files. This operation will override any previous configuration.
*
* @param ignoredAttributes the attributes to ignore
* @since 1.15
*/
RuntimeClasspathNormalization.MetaInf setIgnoredAttributes(String... ignoredAttributes);
/**
* Allows ignoring one or more attributes in {@code MANIFEST} files.
* This operation is additive, i.e. it will add the ignored attributes to the previously configured attributes.
*
* @param ignoredAttributes the attributes to ignore
* @since 1.15
*/
RuntimeClasspathNormalization.MetaInf addIgnoredAttributes(List ignoredAttributes);
/**
* Allows ignoring one or more attributes in {@code MANIFEST} files.
* This operation is additive, i.e. it will add the ignored attributes to the previously configured attributes.
*
* @param ignoredAttributes the attributes to ignore
* @since 1.15
*/
RuntimeClasspathNormalization.MetaInf addIgnoredAttributes(String... ignoredAttributes);
/**
* Allows ignoring one or more properties. This operation will override any previous configuration.
*
* @param ignoredProperties the properties to ignore
* @since 1.15
*/
RuntimeClasspathNormalization.MetaInf setIgnoredProperties(List ignoredProperties);
/**
* Allows ignoring one or more properties. This operation will override any previous configuration.
*
* @param ignoredProperties the properties to ignore
* @since 1.15
*/
RuntimeClasspathNormalization.MetaInf setIgnoredProperties(String... ignoredProperties);
/**
* Allows ignoring one or more properties. This operation is additive,
* i.e. it will add the ignored properties to the previously configured properties.
*
* @param ignoredProperties the properties to ignore
* @since 1.15
*/
RuntimeClasspathNormalization.MetaInf addIgnoredProperties(List ignoredProperties);
/**
* Allows ignoring one or more properties. This operation is additive,
* i.e. it will add the ignored properties to the previously configured properties.
*
* @param ignoredProperties the properties to ignore
* @since 1.15
*/
RuntimeClasspathNormalization.MetaInf addIgnoredProperties(String... ignoredProperties);
/**
* Allows ignoring the {@code MANIFEST} files completely.
*
* @param ignoreManifest whether to ignore the {@code MANIFEST} files
* @since 1.15
*/
RuntimeClasspathNormalization.MetaInf setIgnoreManifest(boolean ignoreManifest);
/**
* Allows ignoring all files in the {@code META-INF} directories.
*
* @param ignoreCompletely whether to completely ignore the files in {@code META-INF} directories
* @since 1.15
*/
RuntimeClasspathNormalization.MetaInf setIgnoreCompletely(boolean ignoreCompletely);
}
}
/**
* Allows configuring the system property normalization of a project.
*
* @since 1.18
*/
interface SystemPropertiesNormalization {
/**
* Allows configuring the normalization to ignore system properties supplied.
*
* @param systemPropertyNames the system properties to be ignored
* @since 1.18
*/
SystemPropertiesNormalization setIgnoredKeys(String... systemPropertyNames);
/**
* Allows configuring the normalization to ignore system properties supplied.
*
* @param systemPropertyNames the system properties to be ignored
* @since 1.18
*/
SystemPropertiesNormalization setIgnoredKeys(List systemPropertyNames);
/**
* Allows configuring the normalization to ignore system properties supplied.
*
* @param systemPropertyNames the system properties to be ignored
* @since 1.18
*/
SystemPropertiesNormalization addIgnoredKeys(String... systemPropertyNames);
/**
* Allows configuring the normalization to ignore system properties supplied.
*
* @param systemPropertyNames the system properties to be ignored
* @since 1.18
*/
SystemPropertiesNormalization addIgnoredKeys(List systemPropertyNames);
}
}