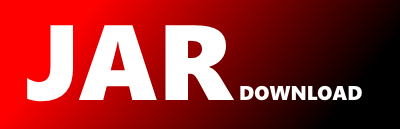
com.gradle.maven.extension.api.scan.BuildScanApi Maven / Gradle / Ivy
Show all versions of develocity-maven-extension Show documentation
package com.gradle.maven.extension.api.scan;
import javax.annotation.Nullable;
import java.net.URI;
import java.util.function.Consumer;
/**
* Allows to interact with the build scan feature of the Gradle Enterprise Maven extension.
*
* @since 1.0
* @deprecated since 1.21, replaced by {@link com.gradle.develocity.agent.maven.api.scan.BuildScanApi}
*/
@Deprecated
public interface BuildScanApi {
/**
* Captures a tag for the current build. The tag is not required to be unique for a given build.
*
* @param tag the name of the tag, must not be null
* @since 1.0
*/
void tag(String tag);
/**
* Captures a named value for the current build. The name is not required to be unique for a given build.
*
* @param name the name, must not be null
* @param value the value, may be null
* @since 1.0
*/
void value(String name, String value);
/**
* Captures a named link for the current build. The name is not required to be unique for a given build.
*
* @param name the name, must not be null
* @param url the url, must not be null, must be a valid valid http:, https: or mailto: URL
* @since 1.0
*/
void link(String name, String url);
/**
* Executes the given action in a background thread, allowing the current Maven work to continue.
*
* This method is useful for capturing values, tags and links that are expensive to compute.
* By capturing them in the background, Maven can continue doing other work, making your build faster.
*
* For example, if you are capturing the Git commit ID as a custom value you should invoke Git in the background:
*
* import org.eclipse.jgit.*;
* background(api -> {
* Git git = ...
* ObjectId objectId = git.getRepository().resolve("HEAD");
* api.value("Git Commit ID", ObjectId.toString(objectId));
* });
*
*
* All background work will be completed before finishing the build and publishing the build scan.
*
* Any errors that are thrown by the background action will be logged and captured in the build scan.
*
* @param action the action to execute in the background
* @since 1.2
*/
void background(Consumer super BuildScanApi> action);
/**
* Registers a callback that is invoked when the build has finished, but before this extension stops
* accepting to be called.
*
* @param action the action to execute when the build has finished
* @since 1.2
*/
void buildFinished(Consumer super BuildResult> action);
/**
* Registers a callback that is invoked when a build scan has been published successfully.
*
* If {@link #isUploadInBackground()} is {@code true}, the callback is invoked as early as possible,
* which is as soon as the Gradle Enterprise server provides a build scan URL, and before the actual upload to the server.
* This means that if the URL is accessed right away, it might not be available yet, as the build scan is still being transmitted and processed by the server.
*
* @param action the action to execute when the build scan has been published successfully
* @since 1.2
*/
void buildScanPublished(Consumer super PublishedBuildScan> action);
/**
* The location of the Gradle Terms of Use that are agreed to when creating a build scan.
*
* Configuration via the {@code gradle.scan.termsOfService.url} system property will always take precedence.
*
* @param termsOfServiceUrl the location of the Gradle Terms of Use
* @since 1.2
*/
void setTermsOfServiceUrl(String termsOfServiceUrl);
/**
* The location of the Gradle Terms of Use that are agreed to when creating a build scan.
*
* @return the location of the Gradle Terms of Use
* @since 1.2
*/
@Nullable
String getTermsOfServiceUrl();
/**
* Indicates whether the Gradle Terms of Use specified under {@link #setTermsOfServiceUrl(String)} are agreed to.
*
* Configuration via the {@code gradle.scan.termsOfService.accept} system property will always take precedence.
*
* @param agree true if agreeing to the Gradle Terms of Use, false otherwise
* @since 1.2
*/
void setTermsOfServiceAgree(String agree);
/**
* The agreement of the Gradle Terms of Use specified under {@link #setTermsOfServiceUrl(String)}.
*
* @return the agreement of the Gradle Terms of Use
* @since 1.2
*/
@Nullable
String getTermsOfServiceAgree();
/**
* Sets the URL of the Gradle Enterprise server to which the build scans are published.
*
* @param url the server URL
* @since 1.2
*/
default void setServer(String url) {
setServer(url == null ? null : URI.create(url));
}
/**
* Sets the URL of the Gradle Enterprise server to which the build scans are published.
*
* @param url the server URL
* @since 1.10.3
*/
void setServer(URI url);
/**
* Returns the URL of the Gradle Enterprise server to which the build scans are published.
*
* @return null when no enterprise server is configured
* @since 1.2
*/
@Nullable
String getServer();
/**
* Specifies whether it is acceptable to communicate with a Gradle Enterprise server using an untrusted SSL certificate.
*
* The default (public) build scan server uses SSL certificates that are trusted by default by standard modern Java environments.
* If you are using a different build scan server via Gradle Enterprise, it may use an untrusted certificate.
* This may be due to the use of an internally provisioned or self-signed certificate.
*
* In such a scenario, you can either configure the build JVM environment to trust the certificate,
* or call this method with {@code true} to disable verification of the server's identity.
* Alternatively, you may disable SSL completely for Gradle Enterprise installation but this is not recommended.
*
* Allowing communication with untrusted servers keeps data encrypted during transmission,
* but makes it easy for a man-in-the-middle to impersonate the intended server and capture data.
*
* This value has no effect if a server is specified using the HTTP protocol (i.e. has SSL disabled).
*
* @param allow whether to allow communication with a HTTPS server with an untrusted certificate
* @since 1.2
*/
void setAllowUntrustedServer(boolean allow);
/**
* Whether it is acceptable to communicate with a build scan server with an untrusted SSL certificate.
*
* @return {@code true} it is acceptable to communicate with a build scan server with an untrusted SSL certificate
* @since 1.2
*/
boolean getAllowUntrustedServer();
/**
* Indicates that a build scan should be published at the end of the build, regardless of whether
* the build succeeded or failed.
*
* @since 1.2
*/
void publishAlways();
/**
* Indicates that, if the given condition is true, a build scan should be published at the end of the build, regardless
* of whether the build succeeded or failed.
*
* @param condition whether to publish
* @since 1.2
*/
void publishAlwaysIf(boolean condition);
/**
* Indicates that a build scan should be published at the end of the build, if and only if the build failed.
*
* @since 1.2
*/
void publishOnFailure();
/**
* Indicates that, if the given condition is true, a build scan should be published at the end of the build, if
* and only if the build failed.
*
* @param condition whether to publish on failure
* @since 1.2
*/
void publishOnFailureIf(boolean condition);
/**
* Indicates that a build scan should be published only if {@code -Dscan} is passed to the Maven invocation.
*
* @since 1.2
*/
void publishOnDemand();
/**
* Specifies whether to upload the build scan in background after the build has finished.
*
* Defaults to {@code true}.
*
* This allows the build to finish sooner, but can be problematic in build environments that
* terminate as soon as the build is finished as the upload may be terminated before it completes.
* Background uploading should be disabled for such environments.
*
* This property may also be set in gradle-enterprise.xml or via the {@code gradle.scan.uploadInBackground} system property,
* which if set takes precedence over any value set by this method and the default.
* If this is set to any value other than {@code true}, the background build scan upload will be disabled.
*
* This method cannot be called after the MavenSession has been started (i.e. after the {@link org.apache.maven.execution.ExecutionEvent.Type#SessionStarted} event has been received).
* Doing so will produce a build time error.
*
* @param uploadInBackground whether to upload the build scan in background
* @since 1.5
*/
void setUploadInBackground(boolean uploadInBackground);
/**
* See {@link #setUploadInBackground(boolean)}.
*
* @return whether to upload build scan in background
* @since 1.5
*/
boolean isUploadInBackground();
/**
* Specifies whether to capture information about each file used as an input to a goal execution.
*
* Defaults to {@code false}.
*
* Enabling this feature may increase the size of the build scan data.
* This requires more time to transmit to the server, and more storage space at the server.
* Most builds will not incur a noticeable difference when this feature is enabled.
* Large builds may increase the build scan data by a handful of megabytes.
* For most builds, the increase will be negligible.
*
* If using Gradle Enterprise with a good connection to the server this capture should be enabled,
* as it allows comparing goal inputs at a file level when comparing builds.
*
* This property may also be set in gradle-enterprise.xml or via the {@code gradle.scan.captureGoalInputFiles} system property.
* If this is set to any value other than {@code false}, the capture will be enabled.
* If this is set to {@code false}, the capture will be disabled.
* If the capture is enabled or disabled via system property, calling this method has no effect.
* That is, the system property takes precedence over the value set via this method.
*
* This method cannot be called after the MavenSession has been started (i.e. after the {@link org.apache.maven.execution.ExecutionEvent.Type#SessionStarted} event has been received).
* Doing so will produce a build time error.
*
* @param capture whether to capture information about each file use as an input to a goal execution
* @since 1.2
* @deprecated Please use {@link BuildScanCaptureSettings#setGoalInputFiles(boolean)}
*/
@Deprecated
void setCaptureGoalInputFiles(boolean capture);
/**
* See {@link #setCaptureGoalInputFiles(boolean)}.
*
* @return whether information about each file used as an input to a goal will be captured
* @since 1.2
* @deprecated Please use {@link BuildScanCaptureSettings#isGoalInputFiles()}
*/
@Deprecated
boolean isCaptureGoalInputFiles();
/**
* Executes the given action only once over the course of the Maven build execution.
*
* The identifier is used to uniquely identify a given action.
* Calling this API a second time with the same identifier will not run the action again.
*
* Any errors that are thrown by the action will be logged and captured in the build scan. In case of a failure while executing the action for a given identifier, no other action will be executed for the same identifier.
*
* @param identifier a unique identifier
* @param action the action to execute only once
* @since 1.2.3
*/
void executeOnce(String identifier, Consumer super BuildScanApi> action);
/**
* Allows registering functions for obfuscating certain identifying information within build scans.
*
* @return the register of obfuscation functions
* @since 1.3.1
*/
BuildScanDataObfuscation getObfuscation();
/**
* Allows registering functions for obfuscating certain identifying information within build scans.
*
* @param action a function to be applied to the register of obfuscation functions
* @since 1.3.1
*/
void obfuscation(Consumer super BuildScanDataObfuscation> action);
/**
* Allows configuring what data will be captured as part of the build scan.
*
* @return the capture settings
* @since 1.11
*/
BuildScanCaptureSettings getCapture();
/**
* Allows configuring what data will be captured as part of the build scan.
*
* @param action a function to be applied to the capture settings
* @since 1.11
*/
void capture(Consumer super BuildScanCaptureSettings> action);
}