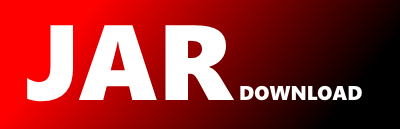
com.graphaware.test.integration.DatabaseIntegrationTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tests Show documentation
Show all versions of tests Show documentation
Tools for testing Neo4j-related and GraphAware-related code
/*
* Copyright (c) 2015 GraphAware
*
* This file is part of GraphAware.
*
* GraphAware is free software: you can redistribute it and/or modify it under the terms of
* the GNU General Public License as published by the Free Software Foundation, either
* version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY;
* without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the GNU General Public License for more details. You should have received a copy of
* the GNU General Public License along with this program. If not, see
* .
*/
package com.graphaware.test.integration;
import com.graphaware.test.data.DatabasePopulator;
import org.junit.After;
import org.junit.Before;
import org.neo4j.graphdb.GraphDatabaseService;
import org.neo4j.graphdb.Transaction;
import org.neo4j.graphdb.factory.GraphDatabaseBuilder;
import org.neo4j.test.TestGraphDatabaseFactory;
import static com.graphaware.common.util.DatabaseUtils.registerShutdownHook;
/**
* Base class for all kinds of Neo4j integration tests.
*
* Creates an {@link org.neo4j.test.ImpermanentGraphDatabase} (by default) at the beginning of each test and allows
* subclasses to populate it by overriding the {@link #populateDatabase(org.neo4j.graphdb.GraphDatabaseService)} method,
* or by providing a {@link com.graphaware.test.data.DatabasePopulator} by overriding the {@link #databasePopulator()} method.
*
* Shuts the database down at the end of each test.
*/
public abstract class DatabaseIntegrationTest {
private GraphDatabaseService database;
@Before
public void setUp() throws Exception {
database = createDatabase();
populateDatabase(database);
}
@After
public void tearDown() throws Exception {
database.shutdown();
}
/**
* Instantiate a database. By default this will be {@link org.neo4j.test.ImpermanentGraphDatabase}.
*
* @return new database.
*/
protected GraphDatabaseService createDatabase() {
GraphDatabaseBuilder graphDatabaseBuilder = new TestGraphDatabaseFactory().newImpermanentDatabaseBuilder();
if (propertiesFile() != null) {
graphDatabaseBuilder = graphDatabaseBuilder.loadPropertiesFromFile(propertiesFile());
}
GraphDatabaseService database = graphDatabaseBuilder.newGraphDatabase();
registerShutdownHook(database);
return database;
}
/**
* Get the name of properties file used to configure the database.
*
* @return properties file, null
for none.
*/
protected String propertiesFile() {
return null;
}
/**
* Populate the database. Can be overridden. By default, it populates the database using {@link #databasePopulator()}.
*
* @param database to populate.
*/
protected void populateDatabase(GraphDatabaseService database) {
DatabasePopulator populator = databasePopulator();
if (populator != null) {
populator.populate(database);
}
}
/**
* @return {@link com.graphaware.test.data.DatabasePopulator}, null
(no population) by default.
*/
protected DatabasePopulator databasePopulator() {
return null;
}
/**
* Get the database instantiated for this test.
*
* @return database.
*/
protected GraphDatabaseService getDatabase() {
return database;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy