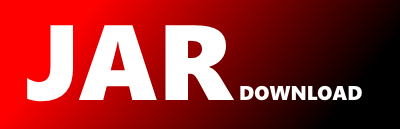
com.graphaware.reco.generic.util.Assert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of recommendation-engine Show documentation
Show all versions of recommendation-engine Show documentation
Skeleton for a Recommendation Engine
The newest version!
package com.graphaware.reco.generic.util;
import java.util.Collection;
import java.util.Map;
public final class Assert {
private Assert() {
}
/**
* Assert a boolean expression, throwing {@code IllegalArgumentException}
* if the test result is {@code false}.
* Assert.isTrue(i > 0, "The value must be greater than zero");
*
* @param expression a boolean expression
* @param message the exception message to use if the assertion fails
* @throws IllegalArgumentException if expression is {@code false}
*/
public static void isTrue(boolean expression, String message) {
if (!expression) {
throw new IllegalArgumentException(message);
}
}
/**
* Assert a boolean expression, throwing {@code IllegalArgumentException}
* if the test result is {@code false}.
* Assert.isTrue(i > 0);
*
* @param expression a boolean expression
* @throws IllegalArgumentException if expression is {@code false}
*/
public static void isTrue(boolean expression) {
isTrue(expression, "[Assertion failed] - this expression must be true");
}
/**
* Assert that an object is {@code null} .
* Assert.isNull(value, "The value must be null");
*
* @param object the object to check
* @param message the exception message to use if the assertion fails
* @throws IllegalArgumentException if the object is not {@code null}
*/
public static void isNull(Object object, String message) {
if (object != null) {
throw new IllegalArgumentException(message);
}
}
/**
* Assert that an object is {@code null} .
* Assert.isNull(value);
*
* @param object the object to check
* @throws IllegalArgumentException if the object is not {@code null}
*/
public static void isNull(Object object) {
isNull(object, "[Assertion failed] - the object argument must be null");
}
/**
* Assert that an object is not {@code null} .
* Assert.notNull(clazz, "The class must not be null");
*
* @param object the object to check
* @param message the exception message to use if the assertion fails
* @throws IllegalArgumentException if the object is {@code null}
*/
public static void notNull(Object object, String message) {
if (object == null) {
throw new IllegalArgumentException(message);
}
}
/**
* Assert that an object is not {@code null} .
* Assert.notNull(clazz);
*
* @param object the object to check
* @throws IllegalArgumentException if the object is {@code null}
*/
public static void notNull(Object object) {
notNull(object, "[Assertion failed] - this argument is required; it must not be null");
}
/**
* Assert that the given String is not empty; that is,
* it must not be {@code null} and not the empty String.
* Assert.hasLength(name, "Name must not be empty");
*
* @param text the String to check
* @param message the exception message to use if the assertion fails
* @throws IllegalArgumentException if the text is empty
*/
public static void hasLength(String text, String message) {
if ((text != null && text.length() > 0)) {
return;
}
throw new IllegalArgumentException(message);
}
/**
* Assert that the given String is not empty; that is,
* it must not be {@code null} and not the empty String.
* Assert.hasLength(name);
*
* @param text the String to check
* @throws IllegalArgumentException if the text is empty
*/
public static void hasLength(String text) {
hasLength(text,
"[Assertion failed] - this String argument must have length; it must not be null or empty");
}
/**
* Assert that an array has no null elements.
* Note: Does not complain if the array is empty!
* Assert.noNullElements(array, "The array must have non-null elements");
*
* @param array the array to check
* @param message the exception message to use if the assertion fails
* @throws IllegalArgumentException if the object array contains a {@code null} element
*/
public static void noNullElements(Object[] array, String message) {
if (array != null) {
for (Object element : array) {
if (element == null) {
throw new IllegalArgumentException(message);
}
}
}
}
/**
* Assert a boolean expression, throwing {@code IllegalStateException}
* if the test result is {@code false}. Call isTrue if you wish to
* throw IllegalArgumentException on an assertion failure.
* Assert.state(id == null, "The id property must not already be initialized");
*
* @param expression a boolean expression
* @param message the exception message to use if the assertion fails
* @throws IllegalStateException if expression is {@code false}
*/
public static void state(boolean expression, String message) {
if (!expression) {
throw new IllegalStateException(message);
}
}
/**
* Assert a boolean expression, throwing {@link IllegalStateException}
* if the test result is {@code false}.
* Call {@link #isTrue(boolean)} if you wish to
* throw {@link IllegalArgumentException} on an assertion failure.
*
Assert.state(id == null);
*
* @param expression a boolean expression
* @throws IllegalStateException if the supplied expression is {@code false}
*/
public static void state(boolean expression) {
state(expression, "[Assertion failed] - this state invariant must be true");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy