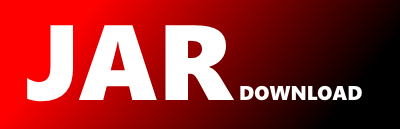
com.graphhopper.api.GHMRequest Maven / Gradle / Ivy
The newest version!
package com.graphhopper.api;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.graphhopper.util.PMap;
import com.graphhopper.util.shapes.GHPoint;
import java.util.Collections;
import java.util.List;
/**
* @author Peter Karich
*/
public class GHMRequest {
private String profile;
private List points;
private List fromPoints;
private List toPoints;
private List pointHints;
private List fromPointHints;
private List toPointHints;
private List curbsides;
private List fromCurbsides;
private List toCurbsides;
private List snapPreventions;
private final PMap hints = new PMap();
private List outArrays = Collections.EMPTY_LIST;
private boolean failFast = true;
public GHMRequest setProfile(String profile) {
this.profile = profile;
return this;
}
public String getProfile() {
return profile;
}
public GHMRequest setPoints(List points) {
this.points = points;
return this;
}
public List getPoints() {
return points;
}
public GHMRequest setFromPoints(List fromPoints) {
this.fromPoints = fromPoints;
return this;
}
public List getFromPoints() {
return fromPoints;
}
public GHMRequest setToPoints(List toPoints) {
this.toPoints = toPoints;
return this;
}
public List getToPoints() {
return toPoints;
}
public GHMRequest setPointHints(List pointHints) {
this.pointHints = pointHints;
return this;
}
public List getPointHints() {
return pointHints;
}
public GHMRequest setFromPointHints(List fromPointHints) {
this.fromPointHints = fromPointHints;
return this;
}
public List getFromPointHints() {
return fromPointHints;
}
public GHMRequest setToPointHints(List toPointHints) {
this.toPointHints = toPointHints;
return this;
}
public List getToPointHints() {
return toPointHints;
}
public GHMRequest setCurbsides(List curbsides) {
this.curbsides = curbsides;
return this;
}
public List getCurbsides() {
return curbsides;
}
public GHMRequest setFromCurbsides(List fromCurbsides) {
this.fromCurbsides = fromCurbsides;
return this;
}
public List getFromCurbsides() {
return fromCurbsides;
}
public GHMRequest setToCurbsides(List toCurbsides) {
this.toCurbsides = toCurbsides;
return this;
}
public List getToCurbsides() {
return toCurbsides;
}
public GHMRequest setSnapPreventions(List snapPreventions) {
this.snapPreventions = snapPreventions;
return this;
}
public List getSnapPreventions() {
return snapPreventions;
}
// a good trick to serialize unknown properties into the HintsMap
@JsonAnySetter
public GHMRequest putHint(String fieldName, Object value) {
hints.putObject(fieldName, value);
return this;
}
public PMap getHints() {
return hints;
}
/**
* Possible values are 'weights', 'times', 'distances'
*/
public GHMRequest setOutArrays(List outArrays) {
this.outArrays = outArrays;
return this;
}
public List getOutArrays() {
return outArrays;
}
/**
* @param failFast if false the matrix calculation will be continued even when some points are not connected
*/
@JsonProperty("fail_fast")
public void setFailFast(boolean failFast) {
this.failFast = failFast;
}
public boolean getFailFast() {
return failFast;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy