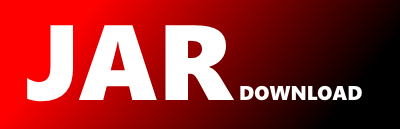
com.graphhopper.api.GHMRequest Maven / Gradle / Ivy
package com.graphhopper.api;
import com.graphhopper.GHRequest;
import com.graphhopper.util.shapes.GHPoint;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
/**
* @author Peter Karich
*/
public class GHMRequest extends GHRequest {
private List fromPoints;
private List toPoints;
boolean identicalLists = true;
private final Set outArrays = new HashSet(5);
public GHMRequest() {
this(10);
}
public GHMRequest(int size) {
super(0);
fromPoints = new ArrayList(size);
toPoints = new ArrayList(size);
}
/**
* Currently: weights, times, distances and paths possible. Where paths is
* the most expensive to calculate and limited to maximum 10*10 points (via
* API end point).
*/
public void addOutArray(String type) {
outArrays.add(type);
}
public Set getOutArrays() {
return outArrays;
}
public GHMRequest addAllPoints(List points) {
for (GHPoint p : points) {
addPoint(p);
}
return this;
}
@Override
public List getPoints() {
throw new IllegalStateException("use getFromPlaces or getToPlaces");
}
public List getFromPoints() {
return fromPoints;
}
public List getToPoints() {
return toPoints;
}
/**
* This methods adds the places as 'from' and 'to' place to the request.
*/
@Override
public GHMRequest addPoint(GHPoint point) {
fromPoints.add(point);
toPoints.add(point);
return this;
}
public GHMRequest addFromPoint(GHPoint point) {
fromPoints.add(point);
identicalLists = false;
return this;
}
public GHMRequest addFromPoints(List points) {
fromPoints = points;
identicalLists = false;
return this;
}
public GHMRequest addToPoint(GHPoint point) {
toPoints.add(point);
identicalLists = false;
return this;
}
public GHMRequest addToPoints(List points) {
toPoints = points;
identicalLists = false;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy