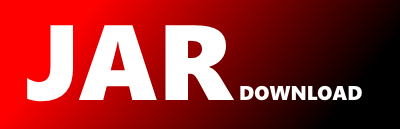
org.allGraphQLCases.client.pojo.AllFieldCases Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of graphql-maven-plugin-samples-allGraphQLCases-pojo-client Show documentation
Show all versions of graphql-maven-plugin-samples-allGraphQLCases-pojo-client Show documentation
This module do integration tests for the generatePojo goal, in client mode
The newest version!
/** Generated by the default template from graphql-java-generator */
package org.allGraphQLCases.client.pojo;
import java.util.HashMap;
import java.util.Map;
import com.graphql_java_generator.annotation.GraphQLInputParameters;
import com.graphql_java_generator.annotation.GraphQLNonScalar;
import com.graphql_java_generator.annotation.GraphQLObjectType;
import com.graphql_java_generator.annotation.GraphQLScalar;
import java.util.List;
import com.graphql_java_generator.annotation.GraphQLDirective;
/**
*
* This type is a clone of the InterfaceAllFieldCases interface.
* Both should contains all possible combinations of parameters, data type, list, mandatory field or items...
*
* @author generated by graphql-java-generator
* @see https://github.com/graphql-java-generator/graphql-java-generator
*/
@GraphQLObjectType("AllFieldCases")
@GraphQLDirective(name = "@testDirective", parameterNames = {"value"}, parameterTypes = {"String!"}, parameterValues = {"on Object\n With a line feed\\\n and a carriage return.\n It also contains 'strange' characters, to check the plugin behavior: \\'\"}])({[\\"})
@GraphQLDirective(name = "@anotherTestDirective")
@GraphQLDirective(name = "@testExtendKeyword", parameterNames = {"msg"}, parameterTypes = {"String"}, parameterValues = {"comes from type extension"})
@SuppressWarnings("unused")
public class AllFieldCases
implements WithID, interfaceToTestExtendKeyword{
/**
* This map contains the deserialized values for the alias, as parsed from the json response from the GraphQL
* server. The key is the alias name, the value is the deserialiazed value (taking into account custom scalars,
* lists, ...)
*/
@com.graphql_java_generator.annotation.GraphQLIgnore
Map aliasValues = new HashMap<>();
public AllFieldCases(){
// No action
}
@GraphQLScalar( fieldName = "id", graphQLTypeSimpleName = "ID", javaClass = java.lang.String.class, listDepth = 0)
@GraphQLDirective(name = "@testDirective", parameterNames = {"value"}, parameterTypes = {"String!"}, parameterValues = {"on Field"})
java.lang.String id;
@GraphQLScalar( fieldName = "name", graphQLTypeSimpleName = "String", javaClass = java.lang.String.class, listDepth = 0)
java.lang.String name;
@GraphQLInputParameters(names = {"uppercase", "textToAppendToTheForname"}, types = {"Boolean", "String"}, mandatories = {false, false}, listDepths = {0, 0}, itemsMandatory = {false, false})
@GraphQLScalar( fieldName = "forname", graphQLTypeSimpleName = "String", javaClass = java.lang.String.class, listDepth = 0)
java.lang.String forname;
@GraphQLInputParameters(names = {"if"}, types = {"String"}, mandatories = {true}, listDepths = {0}, itemsMandatory = {false})
@GraphQLScalar( fieldName = "break", graphQLTypeSimpleName = "String", javaClass = java.lang.String.class, listDepth = 0)
java.lang.String _break;
@GraphQLInputParameters(names = {"unit"}, types = {"Unit"}, mandatories = {false}, listDepths = {0}, itemsMandatory = {false})
@GraphQLScalar( fieldName = "age", graphQLTypeSimpleName = "Long", javaClass = java.lang.Long.class, listDepth = 0)
@GraphQLDirective(name = "@deprecated", parameterNames = {"reason"}, parameterTypes = {"String"}, parameterValues = {"This is a test"})
java.lang.Long age;
@GraphQLScalar( fieldName = "aFloat", graphQLTypeSimpleName = "Float", javaClass = java.lang.Double.class, listDepth = 0)
java.lang.Double aFloat;
@GraphQLScalar( fieldName = "date", graphQLTypeSimpleName = "Date", javaClass = java.util.Date.class, listDepth = 0)
java.util.Date date;
@GraphQLScalar( fieldName = "dateTime", graphQLTypeSimpleName = "DateTime", javaClass = java.time.OffsetDateTime.class, listDepth = 0)
java.time.OffsetDateTime dateTime;
@GraphQLScalar( fieldName = "dates", graphQLTypeSimpleName = "Date", javaClass = java.util.Date.class, listDepth = 1)
List dates;
@GraphQLScalar( fieldName = "nbComments", graphQLTypeSimpleName = "Int", javaClass = java.lang.Integer.class, listDepth = 0)
java.lang.Integer nbComments;
@GraphQLScalar( fieldName = "comments", graphQLTypeSimpleName = "String", javaClass = java.lang.String.class, listDepth = 1)
List comments;
@GraphQLScalar( fieldName = "booleans", graphQLTypeSimpleName = "Boolean", javaClass = java.lang.Boolean.class, listDepth = 1)
List booleans;
@GraphQLScalar( fieldName = "aliases", graphQLTypeSimpleName = "String", javaClass = java.lang.String.class, listDepth = 1)
List aliases;
@GraphQLScalar( fieldName = "planets", graphQLTypeSimpleName = "String", javaClass = java.lang.String.class, listDepth = 1)
List planets;
@GraphQLNonScalar( fieldName = "friends", graphQLTypeSimpleName = "Human", javaClass = org.allGraphQLCases.client.pojo.Human.class, listDepth = 1)
List friends;
@GraphQLScalar( fieldName = "matrix", graphQLTypeSimpleName = "Float", javaClass = java.lang.Double.class, listDepth = 2)
List> matrix;
@GraphQLInputParameters(names = {"uppercase"}, types = {"Boolean"}, mandatories = {false}, listDepths = {0}, itemsMandatory = {false})
@GraphQLNonScalar( fieldName = "oneWithIdSubType", graphQLTypeSimpleName = "AllFieldCasesWithIdSubtype", javaClass = org.allGraphQLCases.client.pojo.AllFieldCasesWithIdSubtype.class, listDepth = 0)
org.allGraphQLCases.client.pojo.AllFieldCasesWithIdSubtype oneWithIdSubType;
@GraphQLInputParameters(names = {"nbItems", "date", "dates", "uppercaseName", "textToAppendToTheForname"}, types = {"Long", "Date", "Date", "Boolean", "String"}, mandatories = {true, false, true, false, false}, listDepths = {0, 0, 1, 0, 0}, itemsMandatory = {false, false, false, false, false})
@GraphQLNonScalar( fieldName = "listWithIdSubTypes", graphQLTypeSimpleName = "AllFieldCasesWithIdSubtype", javaClass = org.allGraphQLCases.client.pojo.AllFieldCasesWithIdSubtype.class, listDepth = 1)
@GraphQLDirective(name = "@generateDataLoaderForLists")
List listWithIdSubTypes;
@GraphQLInputParameters(names = {"input"}, types = {"FieldParameterInput"}, mandatories = {false}, listDepths = {0}, itemsMandatory = {false})
@GraphQLNonScalar( fieldName = "oneWithoutIdSubType", graphQLTypeSimpleName = "AllFieldCasesWithoutIdSubtype", javaClass = org.allGraphQLCases.client.pojo.AllFieldCasesWithoutIdSubtype.class, listDepth = 0)
org.allGraphQLCases.client.pojo.AllFieldCasesWithoutIdSubtype oneWithoutIdSubType;
@GraphQLInputParameters(names = {"nbItems", "input", "textToAppendToTheForname"}, types = {"Long", "FieldParameterInput", "String"}, mandatories = {true, false, false}, listDepths = {0, 0, 0}, itemsMandatory = {false, false, false})
@GraphQLNonScalar( fieldName = "listWithoutIdSubTypes", graphQLTypeSimpleName = "AllFieldCasesWithoutIdSubtype", javaClass = org.allGraphQLCases.client.pojo.AllFieldCasesWithoutIdSubtype.class, listDepth = 1)
List listWithoutIdSubTypes;
@GraphQLInputParameters(names = {"inputs"}, types = {"FieldParameterInput"}, mandatories = {false}, listDepths = {1}, itemsMandatory = {true})
@GraphQLNonScalar( fieldName = "issue65", graphQLTypeSimpleName = "AllFieldCasesWithoutIdSubtype", javaClass = org.allGraphQLCases.client.pojo.AllFieldCasesWithoutIdSubtype.class, listDepth = 1)
List issue65;
@GraphQLInputParameters(names = {"input"}, types = {"AllFieldCasesInput"}, mandatories = {false}, listDepths = {1}, itemsMandatory = {false})
@GraphQLNonScalar( fieldName = "issue66", graphQLTypeSimpleName = "AllFieldCases", javaClass = org.allGraphQLCases.client.pojo.AllFieldCases.class, listDepth = 0)
org.allGraphQLCases.client.pojo.AllFieldCases issue66;
@GraphQLScalar( fieldName = "extendedField", graphQLTypeSimpleName = "String", javaClass = java.lang.String.class, listDepth = 0)
java.lang.String extendedField;
@GraphQLScalar( fieldName = "__typename", graphQLTypeSimpleName = "String", javaClass = java.lang.String.class, listDepth = 0)
java.lang.String __typename;
@Override
@GraphQLDirective(name = "@testDirective", parameterNames = {"value"}, parameterTypes = {"String!"}, parameterValues = {"on Field"})
public void setId(java.lang.String id) {
if (id == null || id instanceof java.lang.String) {
this.id = (java.lang.String) id;
} else {
throw new IllegalArgumentException("The given id should be an instance of java.lang.String, but is an instance of "
+ id.getClass().getName());
}
}
@Override
@GraphQLDirective(name = "@testDirective", parameterNames = {"value"}, parameterTypes = {"String!"}, parameterValues = {"on Field"})
public java.lang.String getId() {
return id;
}
public void setName(java.lang.String name) {
this.name = name;
}
public java.lang.String getName() {
return name;
}
public void setForname(java.lang.String forname) {
this.forname = forname;
}
public java.lang.String getForname() {
return forname;
}
public void setBreak(java.lang.String _break) {
this._break = _break;
}
public java.lang.String getBreak() {
return _break;
}
@GraphQLDirective(name = "@deprecated", parameterNames = {"reason"}, parameterTypes = {"String"}, parameterValues = {"This is a test"})
public void setAge(java.lang.Long age) {
this.age = age;
}
@GraphQLDirective(name = "@deprecated", parameterNames = {"reason"}, parameterTypes = {"String"}, parameterValues = {"This is a test"})
public java.lang.Long getAge() {
return age;
}
public void setAFloat(java.lang.Double aFloat) {
this.aFloat = aFloat;
}
public java.lang.Double getAFloat() {
return aFloat;
}
public void setDate(java.util.Date date) {
this.date = date;
}
public java.util.Date getDate() {
return date;
}
public void setDateTime(java.time.OffsetDateTime dateTime) {
this.dateTime = dateTime;
}
public java.time.OffsetDateTime getDateTime() {
return dateTime;
}
public void setDates(List dates) {
this.dates = dates;
}
public List getDates() {
return dates;
}
public void setNbComments(java.lang.Integer nbComments) {
this.nbComments = nbComments;
}
public java.lang.Integer getNbComments() {
return nbComments;
}
public void setComments(List comments) {
this.comments = comments;
}
public List getComments() {
return comments;
}
public void setBooleans(List booleans) {
this.booleans = booleans;
}
public List getBooleans() {
return booleans;
}
public void setAliases(List aliases) {
this.aliases = aliases;
}
public List getAliases() {
return aliases;
}
public void setPlanets(List planets) {
this.planets = planets;
}
public List getPlanets() {
return planets;
}
public void setFriends(List friends) {
this.friends = friends;
}
public List getFriends() {
return friends;
}
public void setMatrix(List> matrix) {
this.matrix = matrix;
}
public List> getMatrix() {
return matrix;
}
public void setOneWithIdSubType(org.allGraphQLCases.client.pojo.AllFieldCasesWithIdSubtype oneWithIdSubType) {
this.oneWithIdSubType = oneWithIdSubType;
}
public org.allGraphQLCases.client.pojo.AllFieldCasesWithIdSubtype getOneWithIdSubType() {
return oneWithIdSubType;
}
@GraphQLDirective(name = "@generateDataLoaderForLists")
public void setListWithIdSubTypes(List listWithIdSubTypes) {
this.listWithIdSubTypes = listWithIdSubTypes;
}
@GraphQLDirective(name = "@generateDataLoaderForLists")
public List getListWithIdSubTypes() {
return listWithIdSubTypes;
}
public void setOneWithoutIdSubType(org.allGraphQLCases.client.pojo.AllFieldCasesWithoutIdSubtype oneWithoutIdSubType) {
this.oneWithoutIdSubType = oneWithoutIdSubType;
}
public org.allGraphQLCases.client.pojo.AllFieldCasesWithoutIdSubtype getOneWithoutIdSubType() {
return oneWithoutIdSubType;
}
public void setListWithoutIdSubTypes(List listWithoutIdSubTypes) {
this.listWithoutIdSubTypes = listWithoutIdSubTypes;
}
public List getListWithoutIdSubTypes() {
return listWithoutIdSubTypes;
}
public void setIssue65(List issue65) {
this.issue65 = issue65;
}
public List getIssue65() {
return issue65;
}
public void setIssue66(org.allGraphQLCases.client.pojo.AllFieldCases issue66) {
this.issue66 = issue66;
}
public org.allGraphQLCases.client.pojo.AllFieldCases getIssue66() {
return issue66;
}
@Override
public void setExtendedField(java.lang.String extendedField) {
if (extendedField == null || extendedField instanceof java.lang.String) {
this.extendedField = (java.lang.String) extendedField;
} else {
throw new IllegalArgumentException("The given extendedField should be an instance of java.lang.String, but is an instance of "
+ extendedField.getClass().getName());
}
}
@Override
public java.lang.String getExtendedField() {
return extendedField;
}
@Override
public void set__typename(java.lang.String __typename) {
if (__typename == null || __typename instanceof java.lang.String) {
this.__typename = (java.lang.String) __typename;
} else {
throw new IllegalArgumentException("The given __typename should be an instance of java.lang.String, but is an instance of "
+ __typename.getClass().getName());
}
}
@Override
public java.lang.String get__typename() {
return __typename;
}
/**
* This method is called during the json deserialization process, by the {@link GraphQLObjectMapper}, each time an
* alias value is read from the json.
*
* @param aliasName
* @param aliasDeserializedValue
*/
public void setAliasValue(String aliasName, Object aliasDeserializedValue) {
aliasValues.put(aliasName, aliasDeserializedValue);
}
/**
* Retrieves the value for the given alias, as it has been received for this object in the GraphQL response.
* This method should not be used for Custom Scalars, as the parser doesn't know if this alias is a custom
* scalar, and which custom scalar to use at deserialization time. In most case, a value will then be provided by
* this method with a basis json deserialization, but this value won't be the proper custom scalar value.
*
* @param alias
* @return
*/
public Object getAliasValue(String alias) {
return aliasValues.get(alias);
}
public String toString() {
return "AllFieldCases {"
+ "id: " + id
+ ", "
+ "name: " + name
+ ", "
+ "forname: " + forname
+ ", "
+ "_break: " + _break
+ ", "
+ "age: " + age
+ ", "
+ "aFloat: " + aFloat
+ ", "
+ "date: " + date
+ ", "
+ "dateTime: " + dateTime
+ ", "
+ "dates: " + dates
+ ", "
+ "nbComments: " + nbComments
+ ", "
+ "comments: " + comments
+ ", "
+ "booleans: " + booleans
+ ", "
+ "aliases: " + aliases
+ ", "
+ "planets: " + planets
+ ", "
+ "friends: " + friends
+ ", "
+ "matrix: " + matrix
+ ", "
+ "oneWithIdSubType: " + oneWithIdSubType
+ ", "
+ "listWithIdSubTypes: " + listWithIdSubTypes
+ ", "
+ "oneWithoutIdSubType: " + oneWithoutIdSubType
+ ", "
+ "listWithoutIdSubTypes: " + listWithoutIdSubTypes
+ ", "
+ "issue65: " + issue65
+ ", "
+ "issue66: " + issue66
+ ", "
+ "extendedField: " + extendedField
+ ", "
+ "__typename: " + __typename
+ "}";
}
public static Builder builder() {
return new Builder();
}
/**
* The Builder that helps building instance of this POJO. You can get an instance of this class, by calling the
* {@link #builder()}
*/
public static class Builder {
private java.lang.String id;
private java.lang.String name;
private java.lang.String forname;
private java.lang.String _break;
private java.lang.Long age;
private java.lang.Double aFloat;
private java.util.Date date;
private java.time.OffsetDateTime dateTime;
private List dates;
private java.lang.Integer nbComments;
private List comments;
private List booleans;
private List aliases;
private List planets;
private List friends;
private List> matrix;
private org.allGraphQLCases.client.pojo.AllFieldCasesWithIdSubtype oneWithIdSubType;
private List listWithIdSubTypes;
private org.allGraphQLCases.client.pojo.AllFieldCasesWithoutIdSubtype oneWithoutIdSubType;
private List listWithoutIdSubTypes;
private List issue65;
private org.allGraphQLCases.client.pojo.AllFieldCases issue66;
private java.lang.String extendedField;
public Builder withId(java.lang.String id) {
this.id = id;
return this;
}
public Builder withName(java.lang.String name) {
this.name = name;
return this;
}
public Builder withForname(java.lang.String forname) {
this.forname = forname;
return this;
}
public Builder withBreak(java.lang.String _break) {
this._break = _break;
return this;
}
public Builder withAge(java.lang.Long age) {
this.age = age;
return this;
}
public Builder withAFloat(java.lang.Double aFloat) {
this.aFloat = aFloat;
return this;
}
public Builder withDate(java.util.Date date) {
this.date = date;
return this;
}
public Builder withDateTime(java.time.OffsetDateTime dateTime) {
this.dateTime = dateTime;
return this;
}
public Builder withDates(List dates) {
this.dates = dates;
return this;
}
public Builder withNbComments(java.lang.Integer nbComments) {
this.nbComments = nbComments;
return this;
}
public Builder withComments(List comments) {
this.comments = comments;
return this;
}
public Builder withBooleans(List booleans) {
this.booleans = booleans;
return this;
}
public Builder withAliases(List aliases) {
this.aliases = aliases;
return this;
}
public Builder withPlanets(List planets) {
this.planets = planets;
return this;
}
public Builder withFriends(List friends) {
this.friends = friends;
return this;
}
public Builder withMatrix(List> matrix) {
this.matrix = matrix;
return this;
}
public Builder withOneWithIdSubType(org.allGraphQLCases.client.pojo.AllFieldCasesWithIdSubtype oneWithIdSubType) {
this.oneWithIdSubType = oneWithIdSubType;
return this;
}
public Builder withListWithIdSubTypes(List listWithIdSubTypes) {
this.listWithIdSubTypes = listWithIdSubTypes;
return this;
}
public Builder withOneWithoutIdSubType(org.allGraphQLCases.client.pojo.AllFieldCasesWithoutIdSubtype oneWithoutIdSubType) {
this.oneWithoutIdSubType = oneWithoutIdSubType;
return this;
}
public Builder withListWithoutIdSubTypes(List listWithoutIdSubTypes) {
this.listWithoutIdSubTypes = listWithoutIdSubTypes;
return this;
}
public Builder withIssue65(List issue65) {
this.issue65 = issue65;
return this;
}
public Builder withIssue66(org.allGraphQLCases.client.pojo.AllFieldCases issue66) {
this.issue66 = issue66;
return this;
}
public Builder withExtendedField(java.lang.String extendedField) {
this.extendedField = extendedField;
return this;
}
public AllFieldCases build() {
AllFieldCases _object = new AllFieldCases();
_object.setId(id);
_object.setName(name);
_object.setForname(forname);
_object.setBreak(_break);
_object.setAge(age);
_object.setAFloat(aFloat);
_object.setDate(date);
_object.setDateTime(dateTime);
_object.setDates(dates);
_object.setNbComments(nbComments);
_object.setComments(comments);
_object.setBooleans(booleans);
_object.setAliases(aliases);
_object.setPlanets(planets);
_object.setFriends(friends);
_object.setMatrix(matrix);
_object.setOneWithIdSubType(oneWithIdSubType);
_object.setListWithIdSubTypes(listWithIdSubTypes);
_object.setOneWithoutIdSubType(oneWithoutIdSubType);
_object.setListWithoutIdSubTypes(listWithoutIdSubTypes);
_object.setIssue65(issue65);
_object.setIssue66(issue66);
_object.setExtendedField(extendedField);
_object.set__typename("AllFieldCases");
return _object;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy