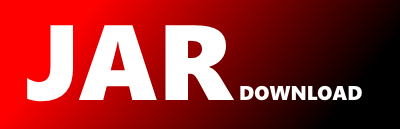
graphql.schema.diffing.Mapping Maven / Gradle / Ivy
package graphql.schema.diffing;
import com.google.common.collect.BiMap;
import com.google.common.collect.HashBiMap;
import graphql.Internal;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
@Internal
public class Mapping {
private BiMap map = HashBiMap.create();
private List sourceList = new ArrayList<>();
private List targetList = new ArrayList<>();
private Mapping(BiMap map, List sourceList, List targetList) {
this.map = map;
this.sourceList = sourceList;
this.targetList = targetList;
}
public Mapping() {
}
public Vertex getSource(Vertex target) {
return map.inverse().get(target);
}
public Vertex getTarget(Vertex source) {
return map.get(source);
}
public Vertex getSource(int i) {
return sourceList.get(i);
}
public Vertex getTarget(int i) {
return targetList.get(i);
}
public List getTargets() {
return targetList;
}
public List getSources() {
return sourceList;
}
public boolean containsSource(Vertex sourceVertex) {
return map.containsKey(sourceVertex);
}
public boolean containsTarget(Vertex targetVertex) {
return map.containsValue(targetVertex);
}
public int size() {
return map.size();
}
public void add(Vertex source, Vertex target) {
this.map.put(source, target);
this.sourceList.add(source);
this.targetList.add(target);
}
public Mapping removeLastElement() {
HashBiMap newMap = HashBiMap.create(map);
newMap.remove(this.sourceList.get(this.sourceList.size() - 1));
List newSourceList = new ArrayList<>(this.sourceList.subList(0, this.sourceList.size() - 1));
List newTargetList = new ArrayList<>(this.targetList.subList(0, this.targetList.size() - 1));
return new Mapping(newMap, newSourceList, newTargetList);
}
public Mapping copy() {
HashBiMap newMap = HashBiMap.create(map);
List newSourceList = new ArrayList<>(this.sourceList);
List newTargetList = new ArrayList<>(this.targetList);
return new Mapping(newMap, newSourceList, newTargetList);
}
public Mapping extendMapping(Vertex source, Vertex target) {
HashBiMap newMap = HashBiMap.create(map);
newMap.put(source, target);
List newSourceList = new ArrayList<>(this.sourceList);
newSourceList.add(source);
List newTargetList = new ArrayList<>(this.targetList);
newTargetList.add(target);
return new Mapping(newMap, newSourceList, newTargetList);
}
public BiMap getMap() {
return map;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Mapping mapping = (Mapping) o;
return Objects.equals(map, mapping.map);
}
@Override
public int hashCode() {
return Objects.hash(map);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy