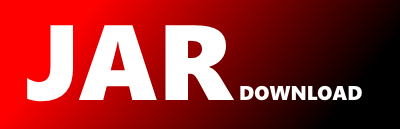
graphql.execution.instrumentation.ChainedInstrumentation Maven / Gradle / Ivy
package graphql.execution.instrumentation;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Maps;
import graphql.Assert;
import graphql.ExecutionInput;
import graphql.ExecutionResult;
import graphql.PublicApi;
import graphql.execution.Async;
import graphql.execution.ExecutionContext;
import graphql.execution.FieldValueInfo;
import graphql.execution.instrumentation.parameters.InstrumentationCreateStateParameters;
import graphql.execution.instrumentation.parameters.InstrumentationExecuteOperationParameters;
import graphql.execution.instrumentation.parameters.InstrumentationExecutionParameters;
import graphql.execution.instrumentation.parameters.InstrumentationExecutionStrategyParameters;
import graphql.execution.instrumentation.parameters.InstrumentationFieldCompleteParameters;
import graphql.execution.instrumentation.parameters.InstrumentationFieldFetchParameters;
import graphql.execution.instrumentation.parameters.InstrumentationFieldParameters;
import graphql.execution.instrumentation.parameters.InstrumentationValidationParameters;
import graphql.language.Document;
import graphql.schema.DataFetcher;
import graphql.schema.GraphQLSchema;
import graphql.validation.ValidationError;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
import java.util.function.Function;
import static graphql.Assert.assertNotNull;
import static graphql.collect.ImmutableKit.mapAndDropNulls;
/**
* This allows you to chain together a number of {@link graphql.execution.instrumentation.Instrumentation} implementations
* and run them in sequence. The list order of instrumentation objects is always guaranteed to be followed and
* the {@link graphql.execution.instrumentation.InstrumentationState} objects they create will be passed back to the originating
* implementation.
*
* @see graphql.execution.instrumentation.Instrumentation
*/
@SuppressWarnings("deprecation")
@PublicApi
public class ChainedInstrumentation implements Instrumentation {
// This class is inspired from https://github.com/leangen/graphql-spqr/blob/master/src/main/java/io/leangen/graphql/GraphQLRuntime.java#L80
protected final ImmutableList instrumentations;
public ChainedInstrumentation(List instrumentations) {
this.instrumentations = ImmutableList.copyOf(assertNotNull(instrumentations));
}
public ChainedInstrumentation(Instrumentation... instrumentations) {
this(Arrays.asList(instrumentations));
}
/**
* @return the list of instrumentations in play
*/
public List getInstrumentations() {
return instrumentations;
}
protected InstrumentationState getSpecificState(Instrumentation instrumentation, InstrumentationState parametersInstrumentationState) {
ChainedInstrumentationState chainedInstrumentationState = (ChainedInstrumentationState) parametersInstrumentationState;
return chainedInstrumentationState.getState(instrumentation);
}
private InstrumentationContext chainedCtx(Function> mapper) {
// if we have zero or 1 instrumentations (and 1 is the most common), then we can avoid an object allocation
// of the ChainedInstrumentationContext since it won't be needed
if (instrumentations.isEmpty()) {
return SimpleInstrumentationContext.noOp();
}
if (instrumentations.size() == 1) {
return mapper.apply(instrumentations.get(0));
}
return new ChainedInstrumentationContext<>(mapAndDropNulls(instrumentations, mapper));
}
@Override
public InstrumentationState createState() {
return Assert.assertShouldNeverHappen("createStateAsync should only ever be used");
}
@Override
public @Nullable InstrumentationState createState(InstrumentationCreateStateParameters parameters) {
return Assert.assertShouldNeverHappen("createStateAsync should only ever be used");
}
@Override
public @NotNull CompletableFuture createStateAsync(InstrumentationCreateStateParameters parameters) {
return ChainedInstrumentationState.combineAll(instrumentations, parameters);
}
@Override
@NotNull
public InstrumentationContext beginExecution(InstrumentationExecutionParameters parameters) {
// these assert methods have been left in so that we truly never call these methods, either in production nor in tests
// later when the deprecated methods are removed, this will disappear.
return Assert.assertShouldNeverHappen("The deprecated " + "beginExecution" + " was called");
}
@Override
public InstrumentationContext beginExecution(InstrumentationExecutionParameters parameters, InstrumentationState state) {
return chainedCtx(instrumentation -> {
InstrumentationState specificState = getSpecificState(instrumentation, state);
return instrumentation.beginExecution(parameters, specificState);
});
}
@Override
@NotNull
public InstrumentationContext beginParse(InstrumentationExecutionParameters parameters) {
return Assert.assertShouldNeverHappen("The deprecated " + "beginParse" + " was called");
}
@Override
public InstrumentationContext beginParse(InstrumentationExecutionParameters parameters, InstrumentationState state) {
return chainedCtx(instrumentation -> {
InstrumentationState specificState = getSpecificState(instrumentation, state);
return instrumentation.beginParse(parameters, specificState);
});
}
@Override
@NotNull
public InstrumentationContext> beginValidation(InstrumentationValidationParameters parameters) {
return Assert.assertShouldNeverHappen("The deprecated " + "beginValidation" + " was called");
}
@Override
public InstrumentationContext> beginValidation(InstrumentationValidationParameters parameters, InstrumentationState state) {
return chainedCtx(instrumentation -> {
InstrumentationState specificState = getSpecificState(instrumentation, state);
return instrumentation.beginValidation(parameters, specificState);
});
}
@Override
@NotNull
public InstrumentationContext beginExecuteOperation(InstrumentationExecuteOperationParameters parameters) {
return Assert.assertShouldNeverHappen("The deprecated " + "beginExecuteOperation" + " was called");
}
@Override
public InstrumentationContext beginExecuteOperation(InstrumentationExecuteOperationParameters parameters, InstrumentationState state) {
return chainedCtx(instrumentation -> {
InstrumentationState specificState = getSpecificState(instrumentation, state);
return instrumentation.beginExecuteOperation(parameters, specificState);
});
}
@Override
@NotNull
public ExecutionStrategyInstrumentationContext beginExecutionStrategy(InstrumentationExecutionStrategyParameters parameters) {
return Assert.assertShouldNeverHappen("The deprecated " + "beginExecutionStrategy" + " was called");
}
@Override
public ExecutionStrategyInstrumentationContext beginExecutionStrategy(InstrumentationExecutionStrategyParameters parameters, InstrumentationState state) {
if (instrumentations.isEmpty()) {
return ExecutionStrategyInstrumentationContext.NOOP;
}
Function mapper = instrumentation -> {
InstrumentationState specificState = getSpecificState(instrumentation, state);
return instrumentation.beginExecutionStrategy(parameters, specificState);
};
if (instrumentations.size() == 1) {
return mapper.apply(instrumentations.get(0));
}
return new ChainedExecutionStrategyInstrumentationContext(mapAndDropNulls(instrumentations, mapper));
}
@Override
@NotNull
public InstrumentationContext beginSubscribedFieldEvent(InstrumentationFieldParameters parameters) {
return Assert.assertShouldNeverHappen("The deprecated " + "beginSubscribedFieldEvent" + " was called");
}
@Override
public InstrumentationContext beginSubscribedFieldEvent(InstrumentationFieldParameters parameters, InstrumentationState state) {
return chainedCtx(instrumentation -> {
InstrumentationState specificState = getSpecificState(instrumentation, state);
return instrumentation.beginSubscribedFieldEvent(parameters, specificState);
});
}
@Override
@NotNull
public InstrumentationContext beginField(InstrumentationFieldParameters parameters) {
return Assert.assertShouldNeverHappen("The deprecated " + "beginField" + " was called");
}
@Override
public InstrumentationContext beginField(InstrumentationFieldParameters parameters, InstrumentationState state) {
return chainedCtx(instrumentation -> {
InstrumentationState specificState = getSpecificState(instrumentation, state);
return instrumentation.beginField(parameters, specificState);
});
}
@Override
@NotNull
public InstrumentationContext
© 2015 - 2025 Weber Informatics LLC | Privacy Policy