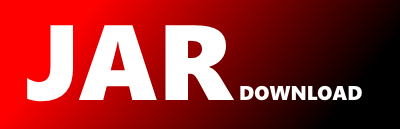
graphql.ExecutionResult Maven / Gradle / Ivy
package graphql;
import java.util.List;
import java.util.Map;
import java.util.function.Consumer;
/**
* This simple value class represents the result of performing a graphql query.
*/
@PublicApi
@SuppressWarnings("TypeParameterUnusedInFormals")
public interface ExecutionResult {
/**
* @return the errors that occurred during execution or empty list if there is none
*/
List getErrors();
/**
* @param allows type coercion
*
* @return the data in the result or null if there is none
*/
T getData();
/**
* The graphql specification specifies:
*
* "If an error was encountered before execution begins, the data entry should not be present in the result.
* If an error was encountered during the execution that prevented a valid response, the data entry in the response should be null."
*
* This allows to distinguish between the cases where {@link #getData()} returns null.
*
* See : https://graphql.github.io/graphql-spec/June2018/#sec-Data
*
* @return true
if the entry "data" should be present in the result
* false
otherwise
*/
boolean isDataPresent();
/**
* @return a map of extensions or null if there are none
*/
Map
© 2015 - 2024 Weber Informatics LLC | Privacy Policy