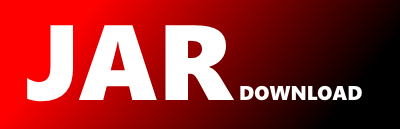
graphql.execution.AbstractAsyncExecutionStrategy Maven / Gradle / Ivy
package graphql.execution;
import com.google.common.collect.Maps;
import graphql.ExecutionResult;
import graphql.ExecutionResultImpl;
import graphql.PublicSpi;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
import java.util.function.BiConsumer;
@PublicSpi
public abstract class AbstractAsyncExecutionStrategy extends ExecutionStrategy {
public AbstractAsyncExecutionStrategy() {
}
public AbstractAsyncExecutionStrategy(DataFetcherExceptionHandler dataFetcherExceptionHandler) {
super(dataFetcherExceptionHandler);
}
protected BiConsumer, Throwable> handleResults(ExecutionContext executionContext, List fieldNames, CompletableFuture overallResult) {
return (List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy