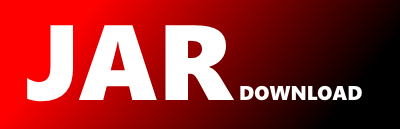
graphql.extensions.DefaultExtensionsMerger Maven / Gradle / Ivy
package graphql.extensions;
import com.google.common.collect.Sets;
import graphql.Internal;
import org.jetbrains.annotations.NotNull;
import java.util.ArrayList;
import java.util.Collection;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
@Internal
public class DefaultExtensionsMerger implements ExtensionsMerger {
@Override
@NotNull
public Map
© 2015 - 2024 Weber Informatics LLC | Privacy Policy