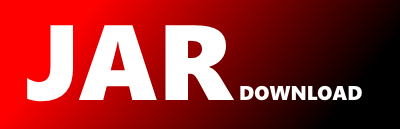
graphql.i18n.I18nMsg Maven / Gradle / Ivy
package graphql.i18n;
import java.util.ArrayList;
import java.util.List;
import static java.util.Arrays.asList;
/**
* A class that represents the intention to create a I18n message
*/
public class I18nMsg {
private final String msgKey;
private final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy