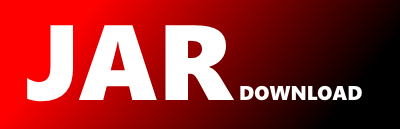
graphql.incremental.IncrementalPayload Maven / Gradle / Ivy
package graphql.incremental;
import graphql.ExperimentalApi;
import graphql.GraphQLError;
import graphql.execution.ResultPath;
import org.jetbrains.annotations.Nullable;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import static java.util.stream.Collectors.toList;
/**
* Represents a payload that can be resolved after the initial response.
*/
@ExperimentalApi
public abstract class IncrementalPayload {
private final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy