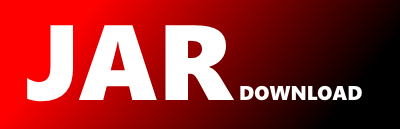
graphql.execution.FieldValueInfo Maven / Gradle / Ivy
package graphql.execution;
import com.google.common.collect.ImmutableList;
import graphql.ExecutionResult;
import graphql.ExecutionResultImpl;
import graphql.PublicApi;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import static graphql.Assert.assertNotNull;
/**
* The {@link FieldValueInfo} holds the type of field that was fetched and completed along with the completed value.
*
* A field value is considered when its is both fetch via a {@link graphql.schema.DataFetcher} to a raw value, and then
* it is serialized into scalar or enum or if it's an object type, it is completed as an object given its field sub selection
*
* The {@link #getFieldValueObject()} method returns either a materialized value or a {@link CompletableFuture}
* promise to a materialized value. Simple in-memory values will tend to be materialized, while complicated
* values might need a call to a database or other systems will tend to be {@link CompletableFuture} promises.
*/
@PublicApi
public class FieldValueInfo {
public enum CompleteValueType {
OBJECT,
LIST,
NULL,
SCALAR,
ENUM
}
private final CompleteValueType completeValueType;
private final Object /* CompletableFuture