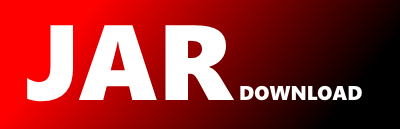
graphql.execution.instrumentation.tracing.TracingSupport Maven / Gradle / Ivy
package graphql.execution.instrumentation.tracing;
import com.google.common.collect.ImmutableList;
import graphql.PublicApi;
import graphql.execution.ExecutionStepInfo;
import graphql.execution.instrumentation.InstrumentationState;
import graphql.schema.DataFetchingEnvironment;
import java.time.Instant;
import java.time.format.DateTimeFormatter;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ConcurrentLinkedQueue;
import static graphql.schema.GraphQLTypeUtil.simplePrint;
/**
* This creates a map of tracing information as outlined in https://github.com/apollographql/apollo-tracing
*
* This is a stateful object that should be instantiated and called via {@link java.lang.instrument.Instrumentation}
* calls. It has been made a separate class so that you can compose this into existing
* instrumentation code.
*/
@PublicApi
public class TracingSupport implements InstrumentationState {
private final Instant startRequestTime;
private final long startRequestNanos;
private final ConcurrentLinkedQueue
© 2015 - 2024 Weber Informatics LLC | Privacy Policy