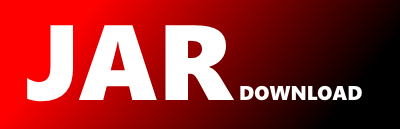
graphql.incremental.DeferPayload Maven / Gradle / Ivy
package graphql.incremental;
import graphql.ExecutionResult;
import graphql.ExperimentalApi;
import graphql.GraphQLError;
import org.jetbrains.annotations.Nullable;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
/**
* Represents a defer payload
*/
@ExperimentalApi
public class DeferPayload extends IncrementalPayload {
private final Object data;
private DeferPayload(Object data, List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy