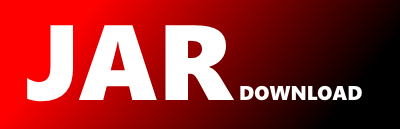
graphql.execution.ExecutionStrategy Maven / Gradle / Ivy
package graphql.execution;
import graphql.ExceptionWhileDataFetching;
import graphql.ExecutionResult;
import graphql.ExecutionResultImpl;
import graphql.GraphQLException;
import graphql.language.Field;
import graphql.schema.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.*;
import static graphql.introspection.Introspection.*;
public abstract class ExecutionStrategy {
private static final Logger log = LoggerFactory.getLogger(ExecutionStrategy.class);
protected ValuesResolver valuesResolver = new ValuesResolver();
protected FieldCollector fieldCollector = new FieldCollector();
public abstract ExecutionResult execute(ExecutionContext executionContext, GraphQLObjectType parentType, Object source, Map> fields);
protected ExecutionResult resolveField(ExecutionContext executionContext, GraphQLObjectType parentType, Object source, List fields) {
GraphQLFieldDefinition fieldDef = getFieldDef(executionContext.getGraphQLSchema(), parentType, fields.get(0));
Map argumentValues = valuesResolver.getArgumentValues(fieldDef.getArguments(), fields.get(0).getArguments(), executionContext.getVariables());
DataFetchingEnvironment environment = new DataFetchingEnvironment(
source,
argumentValues,
executionContext.getRoot(),
fields,
fieldDef.getType(),
parentType,
executionContext.getGraphQLSchema()
);
Object resolvedValue = null;
try {
resolvedValue = fieldDef.getDataFetcher().get(environment);
} catch (Exception e) {
log.info("Exception while fetching data", e);
executionContext.addError(new ExceptionWhileDataFetching(e));
}
return completeValue(executionContext, fieldDef.getType(), fields, resolvedValue);
}
protected ExecutionResult completeValue(ExecutionContext executionContext, GraphQLType fieldType, List fields, Object result) {
if (fieldType instanceof GraphQLNonNull) {
GraphQLNonNull graphQLNonNull = (GraphQLNonNull) fieldType;
ExecutionResult completed = completeValue(executionContext, graphQLNonNull.getWrappedType(), fields, result);
if (completed == null) {
throw new GraphQLException("Cannot return null for non-nullable type: " + fields);
}
return completed;
} else if (result == null) {
return null;
} else if (fieldType instanceof GraphQLList) {
return completeValueForList(executionContext, (GraphQLList) fieldType, fields, result);
} else if (fieldType instanceof GraphQLScalarType) {
return completeValueForScalar((GraphQLScalarType) fieldType, result);
} else if (fieldType instanceof GraphQLEnumType) {
return completeValueForEnum((GraphQLEnumType) fieldType, result);
}
GraphQLObjectType resolvedType;
if (fieldType instanceof GraphQLInterfaceType) {
resolvedType = resolveType((GraphQLInterfaceType) fieldType, result);
} else if (fieldType instanceof GraphQLUnionType) {
resolvedType = resolveType((GraphQLUnionType) fieldType, result);
} else {
resolvedType = (GraphQLObjectType) fieldType;
}
Map> subFields = new LinkedHashMap>();
List visitedFragments = new ArrayList();
for (Field field : fields) {
if (field.getSelectionSet() == null) continue;
fieldCollector.collectFields(executionContext, resolvedType, field.getSelectionSet(), visitedFragments, subFields);
}
// Calling this from the executionContext so that you can shift from the simple execution strategy for mutations
// back to the desired strategy.
return executionContext.getExecutionStrategy().execute(executionContext, resolvedType, result, subFields);
}
private ExecutionResult completeValueForList(ExecutionContext executionContext, GraphQLList fieldType, List fields, Object result) {
if (result.getClass().isArray()) {
result = Arrays.asList((Object[]) result);
}
return completeValueForList(executionContext, fieldType, fields, (List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy