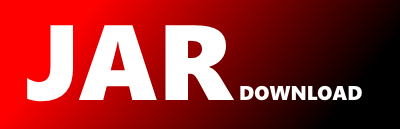
graphql.util.FpKit Maven / Gradle / Ivy
package graphql.util;
import graphql.Internal;
import java.lang.reflect.Array;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.function.BinaryOperator;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
import static java.util.Collections.singletonList;
import static java.util.function.Function.identity;
@Internal
public class FpKit {
//
// From a list of named things, get a map of them by name, merging them according to the merge function
public static Map getByName(List namedObjects, Function nameFn, BinaryOperator mergeFunc) {
return namedObjects.stream().collect(Collectors.toMap(
nameFn,
identity(),
mergeFunc)
);
}
//
// From a list of named things, get a map of them by name, merging them first one added
public static Map getByName(List namedObjects, Function nameFn) {
return getByName(namedObjects, nameFn, mergeFirst());
}
public static BinaryOperator mergeFirst() {
return (o1, o2) -> o1;
}
/**
* Converts an object that should be an Iterable into a Collection efficiently, leaving
* it alone if it is already is one. Useful when you want to get the size of something
*
* @param iterableResult the result object
* @param the type of thing
*
* @return an Iterable from that object
*
* @throws java.lang.ClassCastException if its not an Iterable
*/
@SuppressWarnings("unchecked")
public static Collection toCollection(Object iterableResult) {
if (iterableResult.getClass().isArray()) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy