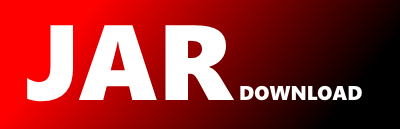
graphql.util.TreeTransformerUtil Maven / Gradle / Ivy
package graphql.util;
import graphql.PublicApi;
import java.util.function.Function;
import static graphql.Assert.assertTrue;
@PublicApi
public class TreeTransformerUtil {
public static TraversalControl changeNode(TraverserContext context, T changedNode) {
NodeZipper zipperWithChangedNode = context.getVar(NodeZipper.class).withNewNode(changedNode);
NodeMultiZipper multiZipper = context.getCurrentAccumulate();
context.setAccumulate(multiZipper.withNewZipper(zipperWithChangedNode));
context.changeNode(changedNode);
return TraversalControl.CONTINUE;
}
public static TraversalControl deleteNode(TraverserContext context) {
NodeZipper curZipper = context.getVar(NodeZipper.class);
NodeAdapter nodeAdaper = context.getVar(NodeAdapter.class);
NodeLocation nodeLocation = curZipper.getBreadcrumbs().get(0).getLocation();
changeParentNode(context, parentNode -> nodeAdaper.removeChild(parentNode, nodeLocation));
context.deleteNode();
return TraversalControl.CONTINUE;
}
public static TraversalControl changeParentNode(TraverserContext context, Function changeNodeFunction) {
assertTrue(context.getParentNode() != null, "can't delete root node");
NodeMultiZipper multiZipper = context.getCurrentAccumulate();
NodeZipper curZipper = context.getVar(NodeZipper.class);
NodeZipper zipperForParent = multiZipper.getZipperForNode(curZipper.getParent());
boolean zipperForParentAlreadyExisted = true;
if (zipperForParent == null) {
zipperForParent = curZipper.moveUp();
zipperForParentAlreadyExisted = false;
}
T parentNode = zipperForParent.getCurNode();
T newParent = changeNodeFunction.apply(parentNode);
NodeZipper newZipperForParent = zipperForParent.withNewNode(newParent);
NodeMultiZipper newMultiZipper;
if (zipperForParentAlreadyExisted) {
newMultiZipper = multiZipper.withReplacedZipper(zipperForParent, newZipperForParent);
} else {
newMultiZipper = multiZipper.withNewZipper(newZipperForParent);
}
context.getParentContext().changeNode(newParent);
context.setAccumulate(newMultiZipper);
return TraversalControl.CONTINUE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy