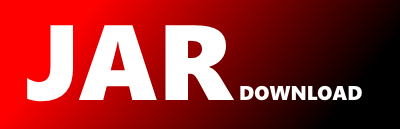
graphql.execution.AbsoluteGraphQLError Maven / Gradle / Ivy
package graphql.execution;
import graphql.ErrorClassification;
import graphql.GraphQLError;
import graphql.Internal;
import graphql.language.SourceLocation;
import graphql.schema.DataFetcher;
import java.util.ArrayList;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.stream.Collectors;
import static graphql.Assert.assertNotNull;
/**
* A {@link GraphQLError} that has been changed from a {@link DataFetcher} relative error to an absolute one.
*/
@Internal
public class AbsoluteGraphQLError implements GraphQLError {
private final List locations;
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy