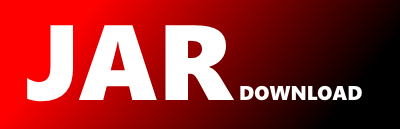
graphql.execution.nextgen.FetchedValueAnalyzer Maven / Gradle / Ivy
package graphql.execution.nextgen;
import graphql.Internal;
import graphql.SerializationError;
import graphql.TypeMismatchError;
import graphql.UnresolvedTypeError;
import graphql.execution.ExecutionContext;
import graphql.execution.ExecutionStepInfo;
import graphql.execution.ExecutionStepInfoFactory;
import graphql.execution.FetchedValue;
import graphql.execution.MergedField;
import graphql.execution.NonNullableFieldWasNullException;
import graphql.execution.ResolveType;
import graphql.execution.UnresolvedTypeException;
import graphql.schema.CoercingSerializeException;
import graphql.schema.GraphQLEnumType;
import graphql.schema.GraphQLObjectType;
import graphql.schema.GraphQLScalarType;
import graphql.schema.GraphQLType;
import graphql.util.FpKit;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import static graphql.execution.nextgen.FetchedValueAnalysis.FetchedValueType.ENUM;
import static graphql.execution.nextgen.FetchedValueAnalysis.FetchedValueType.LIST;
import static graphql.execution.nextgen.FetchedValueAnalysis.FetchedValueType.OBJECT;
import static graphql.execution.nextgen.FetchedValueAnalysis.FetchedValueType.SCALAR;
import static graphql.execution.nextgen.FetchedValueAnalysis.newFetchedValueAnalysis;
import static graphql.schema.GraphQLTypeUtil.isList;
@Internal
public class FetchedValueAnalyzer {
ExecutionStepInfoFactory executionInfoFactory = new ExecutionStepInfoFactory();
ResolveType resolveType = new ResolveType();
/*
* scalar: the value, null and/or error
* enum: same as scalar
* list: list of X: X can be list again, list of scalars or enum or objects
*/
public FetchedValueAnalysis analyzeFetchedValue(ExecutionContext executionContext, FetchedValue fetchedValue, ExecutionStepInfo executionInfo) throws NonNullableFieldWasNullException {
return analyzeFetchedValueImpl(executionContext, fetchedValue, fetchedValue.getFetchedValue(), executionInfo);
}
private FetchedValueAnalysis analyzeFetchedValueImpl(ExecutionContext executionContext, FetchedValue fetchedValue, Object toAnalyze, ExecutionStepInfo executionInfo) throws NonNullableFieldWasNullException {
GraphQLType fieldType = executionInfo.getUnwrappedNonNullType();
MergedField field = executionInfo.getField();
if (isList(fieldType)) {
return analyzeList(executionContext, fetchedValue, toAnalyze, executionInfo);
} else if (fieldType instanceof GraphQLScalarType) {
return analyzeScalarValue(fetchedValue, toAnalyze, (GraphQLScalarType) fieldType, executionInfo);
} else if (fieldType instanceof GraphQLEnumType) {
return analyzeEnumValue(fetchedValue, toAnalyze, (GraphQLEnumType) fieldType, executionInfo);
}
// when we are here, we have a complex type: Interface, Union or Object
// and we must go deeper
//
if (toAnalyze == null) {
return newFetchedValueAnalysis(OBJECT)
.fetchedValue(fetchedValue)
.executionStepInfo(executionInfo)
.nullValue()
.build();
}
try {
GraphQLObjectType resolvedObjectType = resolveType.resolveType(executionContext, field, toAnalyze, executionInfo.getArguments(), fieldType);
return newFetchedValueAnalysis(OBJECT)
.fetchedValue(fetchedValue)
.executionStepInfo(executionInfo)
.completedValue(toAnalyze)
.resolvedType(resolvedObjectType)
.build();
} catch (UnresolvedTypeException ex) {
return handleUnresolvedTypeProblem(fetchedValue, executionInfo, ex);
}
}
private FetchedValueAnalysis handleUnresolvedTypeProblem(FetchedValue fetchedValue, ExecutionStepInfo executionInfo, UnresolvedTypeException e) {
UnresolvedTypeError error = new UnresolvedTypeError(executionInfo.getPath(), executionInfo, e);
return newFetchedValueAnalysis(OBJECT)
.fetchedValue(fetchedValue)
.executionStepInfo(executionInfo)
.nullValue()
.error(error)
.build();
}
private FetchedValueAnalysis analyzeList(ExecutionContext executionContext, FetchedValue fetchedValue, Object toAnalyze, ExecutionStepInfo executionInfo) {
if (toAnalyze == null) {
return newFetchedValueAnalysis(LIST)
.fetchedValue(fetchedValue)
.executionStepInfo(executionInfo)
.nullValue()
.build();
}
if (toAnalyze.getClass().isArray() || toAnalyze instanceof Iterable) {
Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy