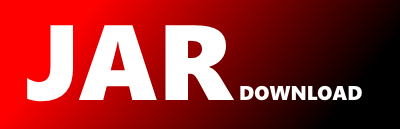
com.graphql.spring.boot.test.RequestFactory Maven / Gradle / Ivy
package com.graphql.spring.boot.test;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.util.LinkedMultiValueMap;
class RequestFactory {
private RequestFactory() {
// utility class
}
static HttpEntity
© 2015 - 2025 Weber Informatics LLC | Privacy Policy