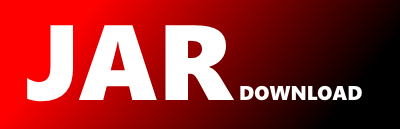
org.dataloader.BatchLoaderEnvironment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-dataloader Show documentation
Show all versions of java-dataloader Show documentation
A pure Java 8 port of Facebook Dataloader
package org.dataloader;
import org.dataloader.annotations.PublicApi;
import org.dataloader.impl.Assertions;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* This object is passed to a batch loader as calling context. It could contain security credentials
* of the calling users for example or database parameters that allow the data layer call to succeed.
*/
@PublicApi
public class BatchLoaderEnvironment {
private final Object context;
private final Map
© 2015 - 2024 Weber Informatics LLC | Privacy Policy