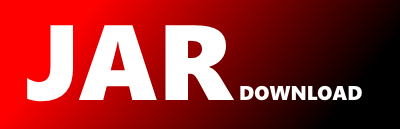
org.dataloader.DataLoaderFactory Maven / Gradle / Ivy
Show all versions of java-dataloader Show documentation
package org.dataloader;
import org.dataloader.annotations.PublicApi;
/**
* A factory class to create {@link DataLoader}s
*/
@SuppressWarnings("unused")
@PublicApi
public class DataLoaderFactory {
/**
* Creates new DataLoader with the specified batch loader function and default options
* (batching, caching and unlimited batch size).
*
* @param batchLoadFunction the batch load function to use
* @param the key type
* @param the value type
*
* @return a new DataLoader
*/
public static DataLoader newDataLoader(BatchLoader batchLoadFunction) {
return newDataLoader(batchLoadFunction, null);
}
/**
* Creates new DataLoader with the specified batch loader function with the provided options
*
* @param batchLoadFunction the batch load function to use
* @param options the options to use
* @param the key type
* @param the value type
*
* @return a new DataLoader
*/
public static DataLoader newDataLoader(BatchLoader batchLoadFunction, DataLoaderOptions options) {
return mkDataLoader(batchLoadFunction, options);
}
/**
* Creates new DataLoader with the specified batch loader function and default options
* (batching, caching and unlimited batch size) where the batch loader function returns a list of
* {@link org.dataloader.Try} objects.
*
* If it's important you to know the exact status of each item in a batch call and whether it threw exceptions then
* you can use this form to create the data loader.
*
* Using Try objects allows you to capture a value returned or an exception that might
* have occurred trying to get a value. .
*
* @param batchLoadFunction the batch load function to use that uses {@link org.dataloader.Try} objects
* @param the key type
* @param the value type
*
* @return a new DataLoader
*/
public static DataLoader newDataLoaderWithTry(BatchLoader> batchLoadFunction) {
return newDataLoaderWithTry(batchLoadFunction, null);
}
/**
* Creates new DataLoader with the specified batch loader function and with the provided options
* where the batch loader function returns a list of
* {@link org.dataloader.Try} objects.
*
* @param batchLoadFunction the batch load function to use that uses {@link org.dataloader.Try} objects
* @param options the options to use
* @param the key type
* @param the value type
*
* @return a new DataLoader
*
* @see #newDataLoaderWithTry(BatchLoader)
*/
public static DataLoader newDataLoaderWithTry(BatchLoader> batchLoadFunction, DataLoaderOptions options) {
return mkDataLoader(batchLoadFunction, options);
}
/**
* Creates new DataLoader with the specified batch loader function and default options
* (batching, caching and unlimited batch size).
*
* @param batchLoadFunction the batch load function to use
* @param the key type
* @param the value type
*
* @return a new DataLoader
*/
public static DataLoader newDataLoader(BatchLoaderWithContext batchLoadFunction) {
return newDataLoader(batchLoadFunction, null);
}
/**
* Creates new DataLoader with the specified batch loader function with the provided options
*
* @param batchLoadFunction the batch load function to use
* @param options the options to use
* @param the key type
* @param the value type
*
* @return a new DataLoader
*/
public static DataLoader newDataLoader(BatchLoaderWithContext batchLoadFunction, DataLoaderOptions options) {
return mkDataLoader(batchLoadFunction, options);
}
/**
* Creates new DataLoader with the specified batch loader function and default options
* (batching, caching and unlimited batch size) where the batch loader function returns a list of
* {@link org.dataloader.Try} objects.
*
* If it's important you to know the exact status of each item in a batch call and whether it threw exceptions then
* you can use this form to create the data loader.
*
* Using Try objects allows you to capture a value returned or an exception that might
* have occurred trying to get a value. .
*
* @param batchLoadFunction the batch load function to use that uses {@link org.dataloader.Try} objects
* @param the key type
* @param the value type
*
* @return a new DataLoader
*/
public static DataLoader newDataLoaderWithTry(BatchLoaderWithContext> batchLoadFunction) {
return newDataLoaderWithTry(batchLoadFunction, null);
}
/**
* Creates new DataLoader with the specified batch loader function and with the provided options
* where the batch loader function returns a list of
* {@link org.dataloader.Try} objects.
*
* @param batchLoadFunction the batch load function to use that uses {@link org.dataloader.Try} objects
* @param options the options to use
* @param the key type
* @param the value type
*
* @return a new DataLoader
*
* @see #newDataLoaderWithTry(BatchLoader)
*/
public static DataLoader newDataLoaderWithTry(BatchLoaderWithContext> batchLoadFunction, DataLoaderOptions options) {
return mkDataLoader(batchLoadFunction, options);
}
/**
* Creates new DataLoader with the specified batch loader function and default options
* (batching, caching and unlimited batch size).
*
* @param batchLoadFunction the batch load function to use
* @param the key type
* @param the value type
*
* @return a new DataLoader
*/
public static DataLoader newMappedDataLoader(MappedBatchLoader batchLoadFunction) {
return newMappedDataLoader(batchLoadFunction, null);
}
/**
* Creates new DataLoader with the specified batch loader function with the provided options
*
* @param batchLoadFunction the batch load function to use
* @param options the options to use
* @param the key type
* @param the value type
*
* @return a new DataLoader
*/
public static DataLoader newMappedDataLoader(MappedBatchLoader batchLoadFunction, DataLoaderOptions options) {
return mkDataLoader(batchLoadFunction, options);
}
/**
* Creates new DataLoader with the specified batch loader function and default options
* (batching, caching and unlimited batch size) where the batch loader function returns a list of
* {@link org.dataloader.Try} objects.
*
* If it's important you to know the exact status of each item in a batch call and whether it threw exceptions then
* you can use this form to create the data loader.
*
* Using Try objects allows you to capture a value returned or an exception that might
* have occurred trying to get a value. .
*
*
* @param batchLoadFunction the batch load function to use that uses {@link org.dataloader.Try} objects
* @param the key type
* @param the value type
*
* @return a new DataLoader
*/
public static DataLoader newMappedDataLoaderWithTry(MappedBatchLoader> batchLoadFunction) {
return newMappedDataLoaderWithTry(batchLoadFunction, null);
}
/**
* Creates new DataLoader with the specified batch loader function and with the provided options
* where the batch loader function returns a list of
* {@link org.dataloader.Try} objects.
*
* @param batchLoadFunction the batch load function to use that uses {@link org.dataloader.Try} objects
* @param options the options to use
* @param the key type
* @param the value type
*
* @return a new DataLoader
*
* @see #newDataLoaderWithTry(BatchLoader)
*/
public static DataLoader newMappedDataLoaderWithTry(MappedBatchLoader> batchLoadFunction, DataLoaderOptions options) {
return mkDataLoader(batchLoadFunction, options);
}
/**
* Creates new DataLoader with the specified mapped batch loader function and default options
* (batching, caching and unlimited batch size).
*
* @param batchLoadFunction the batch load function to use
* @param the key type
* @param the value type
*
* @return a new DataLoader
*/
public static DataLoader newMappedDataLoader(MappedBatchLoaderWithContext batchLoadFunction) {
return newMappedDataLoader(batchLoadFunction, null);
}
/**
* Creates new DataLoader with the specified batch loader function with the provided options
*
* @param batchLoadFunction the batch load function to use
* @param options the options to use
* @param the key type
* @param the value type
*
* @return a new DataLoader
*/
public static DataLoader newMappedDataLoader(MappedBatchLoaderWithContext batchLoadFunction, DataLoaderOptions options) {
return mkDataLoader(batchLoadFunction, options);
}
/**
* Creates new DataLoader with the specified batch loader function and default options
* (batching, caching and unlimited batch size) where the batch loader function returns a list of
* {@link org.dataloader.Try} objects.
*
* If it's important you to know the exact status of each item in a batch call and whether it threw exceptions then
* you can use this form to create the data loader.
*
* Using Try objects allows you to capture a value returned or an exception that might
* have occurred trying to get a value. .
*
* @param batchLoadFunction the batch load function to use that uses {@link org.dataloader.Try} objects
* @param the key type
* @param the value type
*
* @return a new DataLoader
*/
public static DataLoader newMappedDataLoaderWithTry(MappedBatchLoaderWithContext> batchLoadFunction) {
return newMappedDataLoaderWithTry(batchLoadFunction, null);
}
/**
* Creates new DataLoader with the specified batch loader function and with the provided options
* where the batch loader function returns a list of
* {@link org.dataloader.Try} objects.
*
* @param batchLoadFunction the batch load function to use that uses {@link org.dataloader.Try} objects
* @param options the options to use
* @param the key type
* @param the value type
*
* @return a new DataLoader
*
* @see #newDataLoaderWithTry(BatchLoader)
*/
public static DataLoader newMappedDataLoaderWithTry(MappedBatchLoaderWithContext> batchLoadFunction, DataLoaderOptions options) {
return mkDataLoader(batchLoadFunction, options);
}
static DataLoader mkDataLoader(Object batchLoadFunction, DataLoaderOptions options) {
return new DataLoader<>(batchLoadFunction, options);
}
}