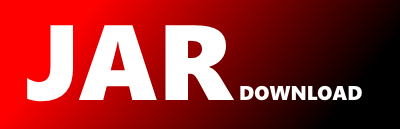
org.dataloader.impl.DefaultCacheMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-dataloader Show documentation
Show all versions of java-dataloader Show documentation
A pure Java 8 port of Facebook Dataloader
/*
* Copyright (c) 2016 The original author or authors
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Apache License v2.0 which accompanies this distribution.
*
* The Eclipse Public License is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* The Apache License v2.0 is available at
* http://www.opensource.org/licenses/apache2.0.php
*
* You may elect to redistribute this code under either of these licenses.
*/
package org.dataloader.impl;
import org.dataloader.CacheMap;
import org.dataloader.annotations.Internal;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
/**
* Default implementation of {@link CacheMap} that is based on a regular {@link java.util.HashMap}.
*
* @param type parameter indicating the type of the cache keys
* @param type parameter indicating the type of the data that is cached
*
* @author Arnold Schrijver
*/
@Internal
public class DefaultCacheMap implements CacheMap {
private final Map> cache;
/**
* Default constructor
*/
public DefaultCacheMap() {
cache = new HashMap<>();
}
/**
* {@inheritDoc}
*/
@Override
public boolean containsKey(K key) {
return cache.containsKey(key);
}
/**
* {@inheritDoc}
*/
@Override
public CompletableFuture get(K key) {
return cache.get(key);
}
/**
* {@inheritDoc}
*/
@Override
public Collection> getAll() {
return cache.values();
}
/**
* {@inheritDoc}
*/
@Override
public CacheMap set(K key, CompletableFuture value) {
cache.put(key, value);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public CacheMap delete(K key) {
cache.remove(key);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public CacheMap clear() {
cache.clear();
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy