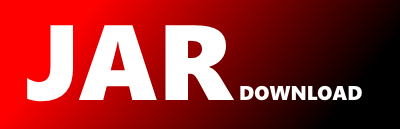
org.dataloader.impl.NoOpValueCache Maven / Gradle / Ivy
Show all versions of java-dataloader Show documentation
package org.dataloader.impl;
import org.dataloader.Try;
import org.dataloader.ValueCache;
import org.dataloader.annotations.Internal;
import java.util.List;
import java.util.concurrent.CompletableFuture;
/**
* Implementation of {@link ValueCache} that does nothing.
*
* We don't want to store values in memory twice, so when using the default store we just
* say we never have the key and complete the other methods by doing nothing.
*
* @param the type of cache keys
* @param the type of cache values
*
* @author Craig Day
*/
@Internal
public class NoOpValueCache implements ValueCache {
/**
* a no op value cache instance
*/
public static final NoOpValueCache, ?> NOOP = new NoOpValueCache<>();
// avoid object allocation by using a final field
private final ValueCachingNotSupported NOT_SUPPORTED = new ValueCachingNotSupported();
private final CompletableFuture NOT_SUPPORTED_CF = CompletableFutureKit.failedFuture(NOT_SUPPORTED);
private final CompletableFuture NOT_SUPPORTED_VOID_CF = CompletableFuture.completedFuture(null);
/**
* {@inheritDoc}
*/
@Override
public CompletableFuture get(K key) {
return NOT_SUPPORTED_CF;
}
@Override
public CompletableFuture>> getValues(List keys) throws ValueCachingNotSupported {
throw NOT_SUPPORTED;
}
/**
* {@inheritDoc}
*/
@Override
public CompletableFuture set(K key, V value) {
return NOT_SUPPORTED_CF;
}
@Override
public CompletableFuture> setValues(List keys, List values) throws ValueCachingNotSupported {
throw NOT_SUPPORTED;
}
/**
* {@inheritDoc}
*/
@Override
public CompletableFuture delete(K key) {
return NOT_SUPPORTED_VOID_CF;
}
/**
* {@inheritDoc}
*/
@Override
public CompletableFuture clear() {
return NOT_SUPPORTED_VOID_CF;
}
}