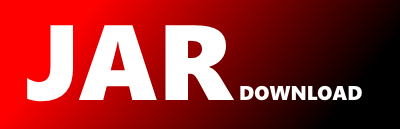
org.dataloader.impl.PromisedValuesImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-dataloader Show documentation
Show all versions of java-dataloader Show documentation
A pure Java 8 port of Facebook Dataloader
package org.dataloader.impl;
import org.dataloader.annotations.Internal;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.CompletionException;
import java.util.concurrent.CompletionStage;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.atomic.AtomicReference;
import java.util.function.Consumer;
import java.util.stream.Collectors;
import static org.dataloader.impl.Assertions.assertState;
import static org.dataloader.impl.Assertions.nonNull;
@Internal
public class PromisedValuesImpl implements PromisedValues {
private final List extends CompletionStage> futures;
private final CompletionStage controller;
private final AtomicReference cause;
private PromisedValuesImpl(List extends CompletionStage> cs) {
this.futures = nonNull(cs);
this.cause = new AtomicReference<>();
CompletableFuture>[] futuresArray = cs.stream().map(CompletionStage::toCompletableFuture).toArray(CompletableFuture[]::new);
this.controller = CompletableFuture.allOf(futuresArray).handle((result, throwable) -> {
setCause(throwable);
return null;
});
}
private PromisedValuesImpl(PromisedValuesImpl other, CompletionStage controller) {
this.futures = other.futures;
this.cause = other.cause;
this.controller = controller;
}
public static PromisedValues combineAllOf(List extends CompletionStage> cfs) {
return new PromisedValuesImpl<>(nonNull(cfs));
}
public static PromisedValues combinePromisedValues(List> promisedValues) {
List> cfs = promisedValues.stream()
.map(pv -> (PromisedValuesImpl) pv)
.flatMap(pv -> pv.futures.stream())
.collect(Collectors.toList());
return new PromisedValuesImpl<>(cfs);
}
private void setCause(Throwable throwable) {
if (throwable != null) {
if (throwable instanceof CompletionException && throwable.getCause() != null) {
cause.set(throwable.getCause());
} else {
cause.set(throwable);
}
}
}
@Override
public PromisedValues thenAccept(Consumer> handler) {
nonNull(handler);
CompletionStage newController = controller.handle((result, throwable) -> {
setCause(throwable);
handler.accept(this);
return result;
});
return new PromisedValuesImpl<>(this, newController);
}
@Override
public boolean succeeded() {
return isDone() && cause.get() == null;
}
@Override
public boolean failed() {
return isDone() && cause.get() != null;
}
@Override
public boolean isDone() {
return controller.toCompletableFuture().isDone();
}
@Override
public Throwable cause() {
return cause.get();
}
@Override
public boolean succeeded(int index) {
return CompletableFutureKit.succeeded(futures.get(index).toCompletableFuture());
}
@Override
public Throwable cause(int index) {
return CompletableFutureKit.cause(futures.get(index).toCompletableFuture());
}
@Override
public T get(int index) {
assertState(isDone(), () -> "The PromisedValues MUST be complete before calling the get() method");
try {
CompletionStage future = futures.get(index);
return future.toCompletableFuture().get();
} catch (InterruptedException | ExecutionException e) {
return null;
}
}
@Override
public List toList() {
assertState(isDone(), () -> "The PromisedValues MUST be complete before calling the toList() method");
int size = size();
List list = new ArrayList<>(size);
for (int index = 0; index < size; index++) {
list.add(get(index));
}
return list;
}
@Override
public int size() {
return futures.size();
}
@Override
public List join() {
controller.toCompletableFuture().join();
return toList();
}
@Override
public CompletableFuture> toCompletableFuture() {
return controller.thenApply(v -> toList()).toCompletableFuture();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy