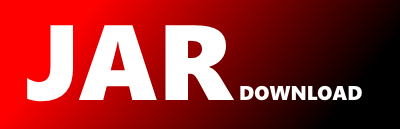
org.dataloader.scheduler.BatchLoaderScheduler Maven / Gradle / Ivy
Show all versions of java-dataloader Show documentation
package org.dataloader.scheduler;
import org.dataloader.BatchLoader;
import org.dataloader.BatchLoaderEnvironment;
import org.dataloader.DataLoader;
import org.dataloader.DataLoaderOptions;
import org.dataloader.MappedBatchLoader;
import java.util.List;
import java.util.Map;
import java.util.concurrent.CompletionStage;
/**
* By default, when {@link DataLoader#dispatch()} is called, the {@link BatchLoader} / {@link MappedBatchLoader} function will be invoked
* immediately. However, you can provide your own {@link BatchLoaderScheduler} that allows this call to be done some time into
* the future. You will be passed a callback ({@link ScheduledBatchLoaderCall} / {@link ScheduledMappedBatchLoaderCall} and you are expected
* to eventually call this callback method to make the batch loading happen.
*
* Note: Because there is a {@link DataLoaderOptions#maxBatchSize()} it is possible for this scheduling to happen N times for a given {@link DataLoader#dispatch()}
* call. The total set of keys will be sliced into batches themselves and then the {@link BatchLoaderScheduler} will be called for
* each batch of keys. Do not assume that a single call to {@link DataLoader#dispatch()} results in a single call to {@link BatchLoaderScheduler}.
*/
public interface BatchLoaderScheduler {
/**
* This represents a callback that will invoke a {@link BatchLoader} function under the covers
*
* @param the value type
*/
interface ScheduledBatchLoaderCall {
CompletionStage> invoke();
}
/**
* This represents a callback that will invoke a {@link MappedBatchLoader} function under the covers
*
* @param the key type
* @param the value type
*/
interface ScheduledMappedBatchLoaderCall {
CompletionStage