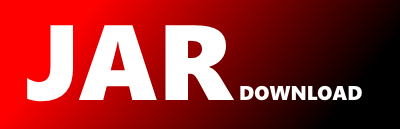
com.greenback.kit.client.GreenbackClient Maven / Gradle / Ivy
package com.greenback.kit.client;
import com.greenback.kit.model.AutoExportQuery;
import com.greenback.kit.model.*;
import java.io.IOException;
public interface GreenbackClient {
String getBaseUrl();
GreenbackCodec getCodec();
// Users
default User getUserById(String userId) throws IOException {
return this.getUserById(userId, null);
}
User getUserById(String userId, Iterable expands) throws IOException;
Entitlements getEntitlements() throws IOException;
Paginated getTeamMembersByTeamId(String teamId) throws IOException;
// Connects
Paginated getConnects(ConnectQuery connectQuery) throws IOException;
default Connect getConnectByLabel(String connectLabel) throws IOException {
return this.getConnectByLabel(connectLabel, null);
}
Connect getConnectByLabel(String connectLabel, Iterable expands) throws IOException;
// Connect Intents
ConnectIntent beginConnectIntent(String connectLabel) throws IOException;
ConnectIntent reconnectAccountIntent(String accountId) throws IOException;
ConnectIntent authorizeConnectIntent(
String token,
ConnectIntentAuthorize authorize) throws IOException;
ConnectIntent completeConnectIntent(
String token,
ConnectIntentComplete complete) throws IOException;
// Accounts
Account createAccount(Account account) throws IOException;
Account updateAccount(Account account) throws IOException;
Paginated getAccounts(AccountQuery accountQuery) throws IOException;
default Account getAccountById(String accountId) throws IOException {
return this.getAccountById(accountId, null);
}
Account getAccountById(String accountId, Iterable expands) throws IOException;
Account deleteAccountById(String accountId) throws IOException;
// Visions
Vision createVision(VisionRequest visionRequest) throws IOException;
default Vision getVisionById(String visionId) throws IOException {
return this.getVisionById(visionId, null);
}
Vision getVisionById(String visionId, Iterable expands) throws IOException;
// Messages
Paginated getMessages(MessageQuery messageQuery) throws IOException;
default Message createMessage(MessageRequest messageRequest) throws IOException {
return this.createMessage(messageRequest, null);
}
Message createMessage(MessageRequest messageRequest, String accountId) throws IOException;
default Message getMessageById(String messageId) throws IOException {
return this.getMessageById(messageId, null);
}
Message getMessageById(String messageId, Iterable expands) throws IOException;
// Transactions
Transaction createTransaction(Transaction transaction) throws IOException;
Transaction updateTransaction(Transaction transaction) throws IOException;
default Transaction getTransactionById(String transactionId) throws IOException {
return this.getTransactionById(transactionId, null);
}
Transaction getTransactionById(String transactionId, Iterable expands) throws IOException;
Paginated getTransactions(TransactionQuery transactionQuery) throws IOException;
Transaction deleteTransactionById(String transactionId) throws IOException;
// Exports
default TransactionExportIntent getTransactionExportIntent(
String transactionId,
String accountId,
TransactionExportIntentRequest transactionExportIntentRequest) throws IOException {
return this.getTransactionExportIntent(transactionId, accountId, null, transactionExportIntentRequest);
}
TransactionExportIntent getTransactionExportIntent(
String transactionId,
String accountId,
String targetId,
TransactionExportIntentRequest transactionExportIntentRequest) throws IOException;
TransactionExport applyTransactionExportIntent(
String transactionId,
String accountId,
TransactionExportIntentRequest transactionExportIntentRequest) throws IOException;
TransactionExport getTransactionExportById(
String transactionExportId) throws IOException;
TransactionExport deleteTransactionExportById(
String transactionExportId,
TransactionExportDeleteMode deleteMode) throws IOException;
// Transforms
Paginated getTransforms(TransformQuery transformQuery) throws IOException;
Transform createTransform(Transform transform) throws IOException;
Transform updateTransform(Transform transform) throws IOException;
Transform getTransformById(String transformId) throws IOException;
Transform deleteTransformById(String transformId, DeleteMode deleteMode) throws IOException;
// Auto Exports
AutoExport createAutoExport(AutoExport autoExport) throws IOException;
AutoExport updateAutoExport(AutoExport autoExport) throws IOException;
Paginated getAutoExports(AutoExportQuery autoExportQuery) throws IOException;
default AutoExport getAutoExportById(String autoExportId) throws IOException {
return this.getAutoExportById(autoExportId, null);
}
AutoExport getAutoExportById(String autoExportId, Iterable expands) throws IOException;
AutoExport deleteAutoExportById(String autoExportId) throws IOException;
// Auto Export Runs
ExportRun createAutoExportRun(String autoExportId, ExportRun exportRun) throws IOException;
default ExportRun getExportRunById(String exportRunId) throws IOException {
return this.getExportRunById(exportRunId, null);
}
ExportRun getExportRunById(String exportRunId, Iterable expands) throws IOException;
default Paginated getExportRunsByAutoExportId(String autoExportId) throws IOException {
return this.getExportRunsByAutoExportId(autoExportId, null);
}
Paginated getExportRunsByAutoExportId(String autoExportId, Iterable expands) throws IOException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy