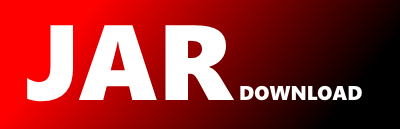
com.greenback.kit.client.impl.ClientHelper Maven / Gradle / Ivy
package com.greenback.kit.client.impl;
import com.fizzed.crux.uri.MutableUri;
import com.greenback.kit.client.GreenbackCodec;
import com.greenback.kit.model.GreenbackException;
import com.greenback.kit.model.Paginated;
import com.greenback.kit.model.Query;
import com.greenback.kit.util.IoFunction;
import com.greenback.kit.util.StreamingPaginated;
import static com.greenback.kit.util.Utils.toStringList;
import java.io.IOException;
import java.time.Instant;
import java.time.ZoneId;
import java.time.format.DateTimeFormatter;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import static java.util.Optional.ofNullable;
import static java.util.stream.Collectors.joining;
import java.util.stream.StreamSupport;
public class ClientHelper {
private static final DateTimeFormatter DTF_ISO_WITH_MILLIS = DateTimeFormatter.ofPattern("yyyy-MM-dd'T'HH:mm:ss.SSS'Z'")
.withZone(ZoneId.of("UTC"));
static public Map toQueryMap(
GreenbackCodec codec,
Object value) throws IOException {
Objects.requireNonNull(codec, "codec was null");
final Map map = new LinkedHashMap<>();
if (value != null) {
final Map flattenedMap = codec.toFlattenedMap(value);
if (flattenedMap != null) {
flattenedMap.forEach((k,v) -> {
map.put(k, ClientHelper.toStringParameter(v));
});
}
}
return map;
}
static public String toStringParameter(Object value) {
if (value instanceof Iterable) {
return toStringList((Iterable)value);
} else if (value instanceof Instant) {
return toInstantParameter((Instant)value);
} else {
return Objects.toString(value, "");
}
}
static public String toInstantParameter(
Instant instant) {
if (instant != null) {
return DTF_ISO_WITH_MILLIS.format(instant);
}
return null;
}
static public Optional toLimitQueryParameter(
Query> query) {
if (query == null) {
return Optional.empty();
}
return ofNullable(query.getLimit());
}
static public Optional toExpandQueryParameter(
Query> query) {
if (query == null) {
return Optional.empty();
}
return ofNullable(toListQueryParameter(query.getExpands()));
}
static public Optional toExpandQueryParameter(
Iterable expands) {
return ofNullable(toListQueryParameter(expands));
}
static public String toListQueryParameter(
Iterable> expands) {
if (expands == null) {
return null;
}
final String param = StreamSupport.stream(expands.spliterator(), false)
.map(v -> Objects.toString(v))
.collect(joining(","));
if (param == null || param.isEmpty()) {
return null;
}
return param;
}
static public T toValue(ClientIoConsumeHandler consumer) throws IOException {
try {
// run the deserializer (it also checks for json-based errors
return consumer.apply();
} catch (GreenbackException e) {
final String category = ofNullable(e.getError())
.map(v -> v.getCategory())
.orElse(null);
if (category != null && "not_found".equalsIgnoreCase(category)) {
return null;
}
throw e; // rethrow it
}
}
static public Paginated toStreamingPaginated(
String url,
IoFunction> method) throws IOException {
Paginated paginated = method.apply(url);
// convert into a streaming version
StreamingPaginated streamingPaginated = new StreamingPaginated<>();
streamingPaginated.setPagination(paginated.getPagination());
streamingPaginated.setValues(paginated.getValues());
// setup streaming pagination
streamingPaginated.nextMethod(v -> {
final String nextUrl = new MutableUri(url)
.setQuery("cursor", v.getNext())
.toString();
return toStreamingPaginated(nextUrl, method);
});
return streamingPaginated;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy