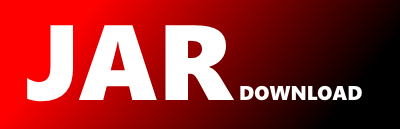
com.greenback.kit.model.Message Maven / Gradle / Ivy
package com.greenback.kit.model;
import java.time.Instant;
import java.util.List;
public class Message extends Document {
private ProcessingStatus status;
private Integer size;
private String subject;
private String snippet;
private List attachments;
private EmailAddress from;
private List replyTo;
private List to;
private List cc;
private List bcc;
private List quoted;
private String fullText;
private Instant postedAt;
// expandable
private List transactions;
public String getName() {
return referenceId;
}
public void setName(String name) {
this.referenceId = name;
}
public ProcessingStatus getStatus() {
return status;
}
public void setStatus(ProcessingStatus status) {
this.status = status;
}
public List getAttachments() {
return attachments;
}
public void setAttachments(List attachments) {
this.attachments = attachments;
}
public Integer getSize() {
return size;
}
public void setSize(Integer size) {
this.size = size;
}
public String getSubject() {
return subject;
}
public void setSubject(String subject) {
this.subject = subject;
}
public String getSnippet() {
return snippet;
}
public void setSnippet(String snippet) {
this.snippet = snippet;
}
public EmailAddress getFrom() {
return from;
}
public void setFrom(EmailAddress from) {
this.from = from;
}
public List getReplyTo() {
return replyTo;
}
public void setReplyTo(List replyTo) {
this.replyTo = replyTo;
}
public List getTo() {
return to;
}
public void setTo(List to) {
this.to = to;
}
public List getCc() {
return cc;
}
public void setCc(List cc) {
this.cc = cc;
}
public List getBcc() {
return bcc;
}
public void setBcc(List bcc) {
this.bcc = bcc;
}
public List getQuoted() {
return quoted;
}
public void setQuoted(List quoted) {
this.quoted = quoted;
}
public String getFullText() {
return fullText;
}
public void setFullText(String fullText) {
this.fullText = fullText;
}
public Instant getPostedAt() {
return postedAt;
}
public void setPostedAt(Instant postedAt) {
this.postedAt = postedAt;
}
public List getTransactions() {
return transactions;
}
public void setTransactions(List transactions) {
this.transactions = transactions;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy