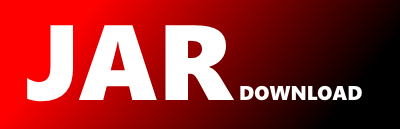
com.greenback.kit.util.Utils Maven / Gradle / Ivy
package com.greenback.kit.util;
import com.greenback.kit.model.Paginated;
import java.util.ArrayList;
import static java.util.Arrays.asList;
import java.util.Collection;
import java.util.HashSet;
import java.util.Objects;
import static java.util.Optional.ofNullable;
import java.util.Set;
public class Utils {
static public String toStringList(Iterable> values) {
final StringBuilder sb = new StringBuilder();
if (values != null) {
for (Object v : values) {
if (sb.length() > 0) {
sb.append(",");
}
sb.append(Objects.toString(v, ""));
}
}
return sb.toString();
}
@SuppressWarnings("unchecked")
static public Iterable appendIterable(Iterable values, T value) {
Objects.requireNonNull(value, "value was null");
if (values == null) {
values = new ArrayList<>();
}
if (values instanceof Collection) {
// add the value
((Collection)values).add(value);
} else {
throw new IllegalArgumentException("Values was not an instanceof a Collection");
}
return values;
}
@SafeVarargs
@SuppressWarnings("varargs")
static public Iterable toIterable(T... values) {
if (values == null) {
return null;
} else {
return asList(values);
}
}
static public Set toSet(Iterable values) {
if (values == null) {
return null;
}
else {
Set newValues = new HashSet<>();
values.forEach(v -> newValues.add(v));
return newValues;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy