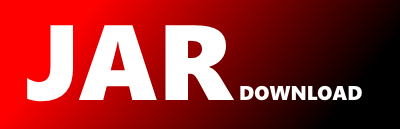
com.greenback.kit.jackson.JacksonGreenbackCodec Maven / Gradle / Ivy
package com.greenback.kit.jackson;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.PropertyNamingStrategy;
import com.fasterxml.jackson.databind.SerializationFeature;
import com.fasterxml.jackson.datatype.jsr310.JavaTimeModule;
import com.fizzed.crux.jackson.EnumDeserializeStrategy;
import com.fizzed.crux.jackson.EnumSerializeStrategy;
import com.fizzed.crux.jackson.EnumStrategyModule;
import com.fizzed.crux.jackson.JavaTimePlusModule;
import com.greenback.kit.client.GreenbackCodec;
import com.greenback.kit.model.*;
import java.io.IOException;
import java.io.InputStream;
import java.text.SimpleDateFormat;
import java.util.Map;
import java.util.TimeZone;
public class JacksonGreenbackCodec implements GreenbackCodec {
protected ObjectMapper objectMapper;
public JacksonGreenbackCodec() {
this.objectMapper = new ObjectMapper()
// .enable(SerializationFeature.INDENT_OUTPUT)
.setSerializationInclusion(Include.NON_NULL)
.disable(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES)
.setPropertyNamingStrategy(PropertyNamingStrategy.SNAKE_CASE)
.disable(SerializationFeature.WRITE_DATES_AS_TIMESTAMPS)
// .registerModule(new JavaTimeModule())
// much more flexible JSR310 de(serializing)
.registerModule(JavaTimePlusModule.build(false))
.registerModule(new EnumStrategyModule(EnumSerializeStrategy.LOWER_CASE, EnumDeserializeStrategy.IGNORE_CASE)
.setNullOnUnknown(true));
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ss.SSSZ");
df.setTimeZone(TimeZone.getTimeZone("UTC"));
this.objectMapper.setDateFormat(df);
}
public ObjectMapper getObjectMapper() {
return objectMapper;
}
public void setObjectMapper(ObjectMapper objectMapper) {
this.objectMapper = objectMapper;
}
protected void verifySuccess(JsonNode rootNode) throws IOException, GreenbackException {
// has error?
if (rootNode.has("error")) {
GreenbackError error = this.objectMapper.treeToValue(rootNode.get("error"), GreenbackError.class);
throw new GreenbackException(error);
}
}
protected T read(
InputStream input,
TypeReference typeReference) throws IOException, GreenbackException {
final JsonNode rootNode = this.objectMapper.readTree(input);
this.verifySuccess(rootNode);
return this.objectMapper.convertValue(rootNode, typeReference);
}
static private final TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy