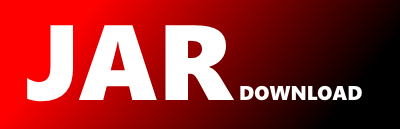
com.greenfiling.smclient.model.AttemptSubmit Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of servemanager-client Show documentation
Show all versions of servemanager-client Show documentation
Java implementation of client for ServeManager API (https://www.servemanager.com/)
/**
* Copyright 2021-2023 Green Filing, LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.greenfiling.smclient.model;
import com.greenfiling.smclient.model.internal.AttemptBase;
public class AttemptSubmit extends AttemptBase {
private String recipientName;
private String recipientDescription;
private String recipientAge;
private String recipientEthnicity;
private String recipientGender;
private String recipientWeight;
private String recipientHeight1;
private String recipientHeight2;
private String recipientHair;
private String recipientRelationship;
private String recipientEyes;
private Integer processServerId;
private Integer addressId;
private Address addressAttributes;
public AttemptSubmit() {
super();
}
public AttemptSubmit(Attempt attempt) {
super(attempt);
// either address_attributes OR addressId can be populated, but not both
if (attempt.getAddress() != null) {
if (attempt.getAddress().getId() != null) {
setAddressId(attempt.getAddress().getId());
} else {
setAddressAttributes(attempt.getAddress());
}
}
// either process_server_id or server_name. Can't be both
if (attempt.getProcessServer() != null) {
if (attempt.getProcessServer().getId() != null) {
setProcessServerId(attempt.getProcessServer().getId());
} else {
setServerName(attempt.getServerName());
}
}
// For some reason the flattened the recipient information instead of using the existing Recipient object
if (attempt.getRecipient() != null) {
setRecipientName(attempt.getRecipient().getName());
setRecipientDescription(attempt.getRecipient().getDescription());
setRecipientAge(attempt.getRecipient().getAge());
setRecipientEthnicity(attempt.getRecipient().getEthnicity());
setRecipientGender(attempt.getRecipient().getGender());
setRecipientWeight(attempt.getRecipient().getWeight());
setRecipientHeight1(attempt.getRecipient().getHeight1());
setRecipientHeight2(attempt.getRecipient().getHeight2());
setRecipientHair(attempt.getRecipient().getHair());
setRecipientRelationship(attempt.getRecipient().getRelationship());
setRecipientEyes(attempt.getRecipient().getEyes());
}
}
public Address getAddressAttributes() {
return this.addressAttributes;
}
public Integer getAddressId() {
return this.addressId;
}
public Integer getProcessServerId() {
return this.processServerId;
}
public String getRecipientAge() {
return this.recipientAge;
}
public String getRecipientDescription() {
return this.recipientDescription;
}
public String getRecipientEthnicity() {
return this.recipientEthnicity;
}
public String getRecipientEyes() {
return this.recipientEyes;
}
public String getRecipientGender() {
return this.recipientGender;
}
public String getRecipientHair() {
return this.recipientHair;
}
public String getRecipientHeight1() {
return this.recipientHeight1;
}
public String getRecipientHeight2() {
return this.recipientHeight2;
}
public String getRecipientName() {
return this.recipientName;
}
public String getRecipientRelationship() {
return this.recipientRelationship;
}
public String getRecipientWeight() {
return this.recipientWeight;
}
public void setAddressAttributes(Address addressAttributes) {
this.addressAttributes = addressAttributes;
}
public void setAddressId(Integer addressId) {
this.addressId = addressId;
}
public void setProcessServerId(Integer processServerId) {
this.processServerId = processServerId;
}
public void setRecipientAge(String recipientAge) {
this.recipientAge = recipientAge;
}
public void setRecipientDescription(String recipientDescription) {
this.recipientDescription = recipientDescription;
}
/**
* Sets the gender
*
* This field uses pre-defined values. Allowed values are:
*
* - {@link Recipient#ETHNICITY_AFRICAN_AMERICAN}
* - {@link Recipient#ETHNICITY_ASIAN_AMERICAN}
* - {@link Recipient#ETHNICITY_CAUCASIAN}
* - {@link Recipient#ETHNICITY_HISPANIC}
* - {@link Recipient#ETHNICITY_LATINO}
* - {@link Recipient#ETHNICITY_MIDDLE_EASTERN}
* - {@link Recipient#ETHNICITY_NATIVE_AMERICAN}
* - {@link Recipient#ETHNICITY_NATIVE_HAWAIIAN}
* - {@link Recipient#ETHNICITY_OTHER}
* - {@link Recipient#ETHNICITY_BLANK}
*
*
* @param recipientEthnicity
* recipient ethnicity
*/
public void setRecipientEthnicity(String recipientEthnicity) {
this.recipientEthnicity = recipientEthnicity;
}
/**
* Sets the eyes field
*
* This field uses pre-defined values. Allowed values are:
*
* - {@link Recipient#EYES_AMBER}
* - {@link Recipient#EYES_BLACK}
* - {@link Recipient#EYES_BLUE}
* - {@link Recipient#EYES_BROWN}
* - {@link Recipient#EYES_GRAY}
* - {@link Recipient#EYES_GREEN}
* - {@link Recipient#EYES_HAZEL}
* - {@link Recipient#EYES_OTHER}
* - {@link Recipient#EYES_BLANK}
*
*
* @param recipientEyes
* recipient eyes
*/
public void setRecipientEyes(String recipientEyes) {
this.recipientEyes = recipientEyes;
}
/**
* Sets the ethnicity
*
* This field uses pre-defined values. Allowed values are:
*
* - {@link Recipient#GENDER_MALE}
* - {@link Recipient#GENDER_FEMALE}
* - {@link Recipient#GENDER_OTHER}
* - {@link Recipient#GENDER_BLANK}
*
*
* @param recipientGender
* recipient gender
*/
public void setRecipientGender(String recipientGender) {
this.recipientGender = recipientGender;
}
/**
* Sets the hair field
*
* This field uses pre-defined values. Allowed values are:
*
* - {@link Recipient#HAIR_BALD}
* - {@link Recipient#HAIR_BLACK}
* - {@link Recipient#HAIR_BLOND}
* - {@link Recipient#HAIR_BROWN}
* - {@link Recipient#HAIR_GRAY}
* - {@link Recipient#HAIR_RED}
* - {@link Recipient#HAIR_WHITE}
* - {@link Recipient#HAIR_OTHER}
* - {@link Recipient#HAIR_BLANK}
*
*
* @param recipientHair
* recipient hair
*/
public void setRecipientHair(String recipientHair) {
this.recipientHair = recipientHair;
}
/**
* Sets the height1 field (the "foot" part of the height)
*
* This field uses pre-defined values. Allowed values are:
*
* - {@link Recipient#HEIGHT_3_FT}
* - {@link Recipient#HEIGHT_4_FT}
* - {@link Recipient#HEIGHT_5_FT}
* - {@link Recipient#HEIGHT_6_FT}
* - {@link Recipient#HEIGHT_7_FT}
* - {@link Recipient#HEIGHT_8_FT}
* - {@link Recipient#HEIGHT_9_FT}
* - {@link Recipient#HEIGHT_BLANK}
*
*
* @param recipientHeight1
* recipient height (feet part)
*/
public void setRecipientHeight1(String recipientHeight1) {
this.recipientHeight1 = recipientHeight1;
}
/**
* Sets the height2 field (the "inch" part of the height)
*
* This field uses pre-defined values. Allowed values are:
*
* - {@link Recipient#HEIGHT_1_IN}
* - {@link Recipient#HEIGHT_2_IN}
* - {@link Recipient#HEIGHT_3_IN}
* - {@link Recipient#HEIGHT_4_IN}
* - {@link Recipient#HEIGHT_5_IN}
* - {@link Recipient#HEIGHT_6_IN}
* - {@link Recipient#HEIGHT_7_IN}
* - {@link Recipient#HEIGHT_8_IN}
* - {@link Recipient#HEIGHT_9_IN}
* - {@link Recipient#HEIGHT_10_IN}
* - {@link Recipient#HEIGHT_11_IN}
* - {@link Recipient#HEIGHT_12_IN}
* - {@link Recipient#HEIGHT_BLANK}
*
*
* @param recipientHeight2
* recipient height (inch part)
*/
public void setRecipientHeight2(String recipientHeight2) {
this.recipientHeight2 = recipientHeight2;
}
public void setRecipientName(String recipientName) {
this.recipientName = recipientName;
}
/**
* Sets the relationship field
*
* This field typically uses pre-defined values, but can use a custom value if desired. The "known" values are
*
* - {@link Recipient#RELATIONSHIP_AUNT}
* - {@link Recipient#RELATIONSHIP_BOYFRIEND}
* - {@link Recipient#RELATIONSHIP_BROTHER}
* - {@link Recipient#RELATIONSHIP_COUSIN}
* - {@link Recipient#RELATIONSHIP_DAUGHTER}
* - {@link Recipient#RELATIONSHIP_FATHER}
* - {@link Recipient#RELATIONSHIP_GIRLFRIEND}
* - {@link Recipient#RELATIONSHIP_GRANDFATHER}
* - {@link Recipient#RELATIONSHIP_GRANDMOTHER}
* - {@link Recipient#RELATIONSHIP_HUSBAND}
* - {@link Recipient#RELATIONSHIP_MOTHER}
* - {@link Recipient#RELATIONSHIP_PARTNER}
* - {@link Recipient#RELATIONSHIP_NEPHEW}
* - {@link Recipient#RELATIONSHIP_NIECE}
* - {@link Recipient#RELATIONSHIP_SISTER}
* - {@link Recipient#RELATIONSHIP_SON}
* - {@link Recipient#RELATIONSHIP_UNCLE}
* - {@link Recipient#RELATIONSHIP_WIFE}
* - {@link Recipient#RELATIONSHIP_OTHER}
* - {@link Recipient#RELATIONSHIP_BLANK}
*
*
* @param recipientRelationship
* recipient relationship
*/
public void setRecipientRelationship(String recipientRelationship) {
this.recipientRelationship = recipientRelationship;
}
public void setRecipientWeight(String recipientWeight) {
this.recipientWeight = recipientWeight;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy