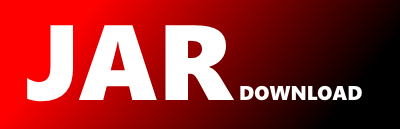
com.greenlaw110.rythm.extension.Transformer Maven / Gradle / Ivy
Show all versions of rythm-engine Show documentation
/*
* Copyright (C) 2013 The Rythm Engine project
* Gelin Luo
*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.greenlaw110.rythm.extension;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
/**
* Transformer
could be used to annotate on a public static methods
* that works on a given object with or without parameters. For example,
*
* @Transformer public static String format(Number number, String formatStr)
*
* When feature.transformer.enabled
is set to true (by default
* it is true), template author can use Transformer to further process an
* expression value:
*
* @args Date dueDate\[email protected]("dd/MM/yyyy")
*
* The @dueDate.format("dd/MM/yyyy")
in the above example will be
* transformed into S.format(dueDate, "dd/MM/yyyy")
in the generated
* java source
*
* Note, the above sample code demonstrates a transformer named format
, which is
* built into Rythm engine. However when you want to define your own transformer, you
* need to use this Transformer
annotation to mark on your methods or classes.
* When the annotation is marked on a class, then all public static methods with return
* value and at least one parameter will be treated as transformer
*
* You can register them to RythmEngine by {@link com.greenlaw110.rythm.RythmEngine#registerTransformer(Class[])}
* method
, once you have registered your Java extension methods, the template author can use them
* in template. Be careful of the name conflict of your Java extension and Rythm's built-in
* Java extension. You can turn off rythm's built java extension by set "rythm.disableBuiltInTransformer"
* to true
*
* Transformers mechanism is also found in other template engine
* solutions, but with different names. Freemarker call it
* built-ins,
* Velocity called it
* velocity tools. But none of them are as easy to use as Rythm Transformers
*/
@Retention(RetentionPolicy.RUNTIME)
@Target({ElementType.METHOD, ElementType.TYPE})
public @interface Transformer {
/**
* The namespace of the transformer. When namespace is presented, the
* template author needs to use the namespace to qualify the transformer
* in the template source. For example, @x.app_myTransformer()
*
* Default value: "app"
*
* @return the namespace
*/
String value() default "app";
/**
* Once specified the pattern will be used to match the token to see if transformer extension
* should be waived or not. For example, @x.escape() should be treated as escape
* transformer while @s().escape(x) shouldn't because s\\(\\) is a waive pattern
*
* @return
*/
String waivePattern() default "";
/**
* Require passing {@link com.greenlaw110.rythm.template.ITemplate template} instance as
* implicit argument (the first parameter)
* @return true if require passing template instance as the implicit first parameter
*/
boolean requireTemplate() default false;
}