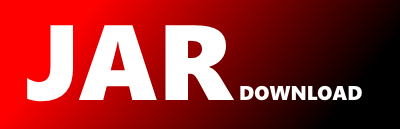
com.greenlaw110.rythm.sandbox.SandboxExecutingService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rythm-engine Show documentation
Show all versions of rythm-engine Show documentation
A strong typed high performance Java Template engine with .Net Razor like syntax
The newest version!
/*
* Copyright (C) 2013 The Rythm Engine project
* Gelin Luo
*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.greenlaw110.rythm.sandbox;
import com.greenlaw110.rythm.RythmEngine;
import com.greenlaw110.rythm.logger.ILogger;
import com.greenlaw110.rythm.logger.Logger;
import com.greenlaw110.rythm.template.ITemplate;
import java.io.File;
import java.util.Map;
import java.util.concurrent.*;
/**
* A secure executing service run template in a separate thread in case there are infinite loop, and also set
* the SecurityManager in the executing thread
*/
public class SandboxExecutingService {
private static ILogger logger = Logger.get(SandboxExecutingService.class);
private ScheduledExecutorService scheduler = null;
private long timeout = 1000;
private RythmEngine engine;
public SandboxExecutingService(int poolSize, SandboxThreadFactory fact, long timeout, RythmEngine re) {
scheduler = new ScheduledThreadPoolExecutor(poolSize, fact, new ThreadPoolExecutor.AbortPolicy());
this.timeout = timeout;
engine = re;
}
private Future
© 2015 - 2024 Weber Informatics LLC | Privacy Policy