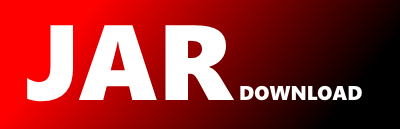
com.groupbyinc.api.model.PartialMatchRule Maven / Gradle / Ivy
package com.groupbyinc.api.model;
import com.groupbyinc.api.interfaces.PartialMatchRuleInterface;
import java.util.Arrays;
/**
* @internal
*/
public class PartialMatchRule implements PartialMatchRuleInterface {
private Integer terms;
private Integer termsGreaterThan;
private Integer mustMatch;
private Boolean percentage;
public Integer getTerms() {
return terms;
}
public PartialMatchRule setTerms(Integer terms) {
this.terms = terms;
return this;
}
public Integer getTermsGreaterThan() {
return termsGreaterThan;
}
public PartialMatchRule setTermsGreaterThan(Integer termsGreaterThan) {
this.termsGreaterThan = termsGreaterThan;
return this;
}
public Integer getMustMatch() {
return mustMatch;
}
public PartialMatchRule setMustMatch(Integer mustMatch) {
this.mustMatch = mustMatch;
return this;
}
public Boolean getPercentage() {
return percentage;
}
public PartialMatchRule setPercentage(Boolean percentage) {
this.percentage = percentage;
return this;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PartialMatchRule that = (PartialMatchRule) o;
return PartialMatchRule.equals(terms, that.terms) && PartialMatchRule.equals(termsGreaterThan, that.termsGreaterThan) && PartialMatchRule.equals(mustMatch, that.mustMatch) && PartialMatchRule.equals(percentage, that.percentage);
}
@Override
public int hashCode() {
return Arrays.hashCode(new Object[] {terms, termsGreaterThan, mustMatch, percentage});
}
/**
* Safe object comparison. TODO: Borrowed from JDK 1.7 and using that for backward compatibility. Should be removed in future.
* Returns {@code true} if the arguments are equal to each other
* and {@code false} otherwise.
* Consequently, if both arguments are {@code null}, {@code true}
* is returned and if exactly one argument is {@code null}, {@code
* false} is returned. Otherwise, equality is determined by using
* the {@link Object#equals equals} method of the first
* argument.
*
* @param a
* @param b
* @return
*/
private static boolean equals(Object a, Object b) {
return (a == b) || (a != null && a.equals(b));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy