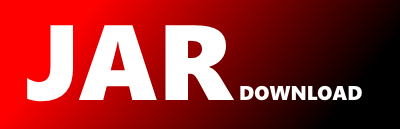
com.groupbyinc.common.test.util.AssertUtils Maven / Gradle / Ivy
package com.groupbyinc.common.test.util;
import org.apache.commons.lang3.StringUtils;
import org.dom4j.DocumentException;
import org.dom4j.io.SAXReader;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import org.skyscreamer.jsonassert.JSONCompare;
import org.skyscreamer.jsonassert.JSONCompareMode;
import org.skyscreamer.jsonassert.JSONCompareResult;
import org.skyscreamer.jsonassert.comparator.DefaultComparator;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.xml.sax.EntityResolver;
import org.xml.sax.InputSource;
import org.xml.sax.SAXException;
import java.io.IOException;
import java.io.StringReader;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import static java.util.Arrays.asList;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertTrue;
import static org.junit.Assert.fail;
public class AssertUtils {
private static final transient Logger LOG = LoggerFactory.getLogger(AssertUtils.class);
public static final int JSON_SPACING = 4;
private AssertUtils() {
// not publicly instantiable
}
public static List grep(String input, String grepFor) {
List result = new ArrayList();
if (StringUtils.isNotBlank(input) && StringUtils.isNotBlank(grepFor)) {
Pattern p = Pattern.compile(grepFor);
for (String line : input.split("\n")) {
Matcher matcher = p.matcher(line);
if (matcher.find()) {
result.add(line);
}
}
}
return result;
}
public static void assertUrlEquals(String lhs, String rhs) {
List list1 = asList(lhs.split("[\\&\\?]"));
List list2 = asList(rhs.split("[\\&\\?]"));
Collections.sort(list1);
Collections.sort(list2);
assertEquals(StringUtils.join(list1, "\n"), StringUtils.join(list2, "\n"));
}
public static void assertListEquals(List expectedLines, List actualLines) {
if (expectedLines.size() != actualLines.size()) {
fail("Expected line count: " + expectedLines.size() + " Actual line count: " + actualLines.size());
}
Collections.sort(expectedLines);
Collections.sort(actualLines);
for (int i = 0; i < expectedLines.size(); i++) {
assertEquals(expectedLines.get(i), actualLines.get(i));
}
}
public static class LenientDoublesJsonComparator extends DefaultComparator {
public static final float MARGIN = 1.0E-06f;
public LenientDoublesJsonComparator() {
super(JSONCompareMode.NON_EXTENSIBLE);
}
@Override
public void compareValues(String prefix, Object expectedValue, Object actualValue, JSONCompareResult result)
throws JSONException {
if (expectedValue instanceof Number && actualValue instanceof Number) {
if (Math.abs(((Number) expectedValue).doubleValue() - ((Number) actualValue).doubleValue()) > MARGIN) {
result.fail(prefix, expectedValue, actualValue);
}
} else {
super.compareValues(prefix, expectedValue, actualValue, result);
}
}
}
public static void assertJsonLinesEquals(List expectedLines, List actualLines) throws Exception {
if (expectedLines.size() != actualLines.size()) {
fail("Expected line count: " + expectedLines.size() + " Actual line count: " + actualLines.size());
}
Collections.sort(expectedLines);
Collections.sort(actualLines);
for (int i = 0; i < expectedLines.size(); i++) {
assertJsonEquals(expectedLines.get(i), actualLines.get(i));
}
}
private static void verifyJson(JSONCompareResult result, String expectedFlat, String expectedFormatted, String actualFlat, String actualFormatted) {
if (!result.passed()) {
LOG.info("reason: {}\nexpected: {}\n actual: {}", result.getMessage(), expectedFlat, actualFlat);
}
assertTrue(
String.format(
"reason: %s\nexpected: %s\n actual: %s", result.getMessage(), expectedFormatted,
actualFormatted), result.passed());
}
public static void assertJsonArrayEquals(String expectedJson, String actualJson) throws JSONException {
JSONArray expected = new JSONArray(expectedJson);
JSONArray actual = new JSONArray(actualJson);
JSONCompareResult result = JSONCompare.compareJSON(expected, actual, new LenientDoublesJsonComparator());
verifyJson(
result, expected.toString().replaceAll("\"", "'"), expected.toString(JSON_SPACING),
actual.toString().replaceAll("\"", "'"), actual.toString(JSON_SPACING));
}
public static void assertJsonEquals(String expectedJson, String actualJson) throws JSONException {
try {
JSONObject expected = new JSONObject(expectedJson);
JSONObject actual = new JSONObject(actualJson);
JSONCompareResult result = JSONCompare.compareJSON(expected, actual, new LenientDoublesJsonComparator());
verifyJson(
result, expected.toString().replaceAll("\"", "'"), expected.toString(JSON_SPACING),
actual.toString().replaceAll("\"", "'"), actual.toString(JSON_SPACING));
} catch (JSONException e) {
LOG.warn("expected: {}\n actual: {}", expectedJson, actualJson);
fail("invalid expected json");
}
}
public static void verifyXmlAgainstDtd(String xml) throws DocumentException {
// verify the file against Google's DTD
SAXReader reader = new SAXReader(true);
reader.setEntityResolver(
new EntityResolver() {
@Override
public InputSource resolveEntity(String publicId, String systemId)
throws SAXException, IOException {
if ("-//Google//DTD GSA Feeds//EN".equals(publicId)) {
return new InputSource(
Thread.currentThread().getContextClassLoader().getResourceAsStream("gsafeed.dtd"));
}
return null;
}
});
reader.read(new StringReader(xml));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy