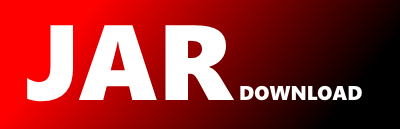
com.groupdocs.sdk.model.AnnotationInfo Maven / Gradle / Ivy
/**
* Copyright 2012 GroupDocs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.groupdocs.sdk.model;
import java.util.*;
import com.groupdocs.sdk.model.Point;
import com.groupdocs.sdk.model.Rectangle;
import com.groupdocs.sdk.model.AnnotationReplyInfo;
import com.groupdocs.sdk.model.Range;
/**
*
*
* NOTE: This class is auto generated by the swagger code generator program. Do not edit the class manually.
*
*/
public class AnnotationInfo {
private String guid = null;
private String documentGuid = null;
private String text = null;
private Long layerId = null;
private String sessionGuid = null;
private String creatorGuid = null;
private String creatorName = null;
private String creatorEmail = null;
private Rectangle box = null;
private Integer pageNumber = null;
private Point annotationPosition = null;
private Range range = null;
private String svgPath = null;
private String type = null;
private String access = null;
private List replies = new ArrayList();
private Long createdOn = null;
private Integer fontColor = null;
private Integer penColor = null;
private Integer penWidth = null;
private Integer penStyle = null;
private Integer backgroundColor = null;
private String fieldText = null;
private String fontFamily = null;
private Double fontSize = null;
public String getGuid() {
return guid;
}
public void setGuid(String guid) {
this.guid = guid;
}
public String getDocumentGuid() {
return documentGuid;
}
public void setDocumentGuid(String documentGuid) {
this.documentGuid = documentGuid;
}
public String getText() {
return text;
}
public void setText(String text) {
this.text = text;
}
public Long getLayerId() {
return layerId;
}
public void setLayerId(Long layerId) {
this.layerId = layerId;
}
public String getSessionGuid() {
return sessionGuid;
}
public void setSessionGuid(String sessionGuid) {
this.sessionGuid = sessionGuid;
}
public String getCreatorGuid() {
return creatorGuid;
}
public void setCreatorGuid(String creatorGuid) {
this.creatorGuid = creatorGuid;
}
public String getCreatorName() {
return creatorName;
}
public void setCreatorName(String creatorName) {
this.creatorName = creatorName;
}
public String getCreatorEmail() {
return creatorEmail;
}
public void setCreatorEmail(String creatorEmail) {
this.creatorEmail = creatorEmail;
}
public Rectangle getBox() {
return box;
}
public void setBox(Rectangle box) {
this.box = box;
}
public Integer getPageNumber() {
return pageNumber;
}
public void setPageNumber(Integer pageNumber) {
this.pageNumber = pageNumber;
}
public Point getAnnotationPosition() {
return annotationPosition;
}
public void setAnnotationPosition(Point annotationPosition) {
this.annotationPosition = annotationPosition;
}
public Range getRange() {
return range;
}
public void setRange(Range range) {
this.range = range;
}
public String getSvgPath() {
return svgPath;
}
public void setSvgPath(String svgPath) {
this.svgPath = svgPath;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getAccess() {
return access;
}
public void setAccess(String access) {
this.access = access;
}
public List getReplies() {
return replies;
}
public void setReplies(List replies) {
this.replies = replies;
}
public Long getCreatedOn() {
return createdOn;
}
public void setCreatedOn(Long createdOn) {
this.createdOn = createdOn;
}
public Integer getFontColor() {
return fontColor;
}
public void setFontColor(Integer fontColor) {
this.fontColor = fontColor;
}
public Integer getPenColor() {
return penColor;
}
public void setPenColor(Integer penColor) {
this.penColor = penColor;
}
public Integer getPenWidth() {
return penWidth;
}
public void setPenWidth(Integer penWidth) {
this.penWidth = penWidth;
}
public Integer getPenStyle() {
return penStyle;
}
public void setPenStyle(Integer penStyle) {
this.penStyle = penStyle;
}
public Integer getBackgroundColor() {
return backgroundColor;
}
public void setBackgroundColor(Integer backgroundColor) {
this.backgroundColor = backgroundColor;
}
public String getFieldText() {
return fieldText;
}
public void setFieldText(String fieldText) {
this.fieldText = fieldText;
}
public String getFontFamily() {
return fontFamily;
}
public void setFontFamily(String fontFamily) {
this.fontFamily = fontFamily;
}
public Double getFontSize() {
return fontSize;
}
public void setFontSize(Double fontSize) {
this.fontSize = fontSize;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AnnotationInfo {\n");
sb.append(" guid: ").append(guid).append("\n");
sb.append(" documentGuid: ").append(documentGuid).append("\n");
sb.append(" text: ").append(text).append("\n");
sb.append(" layerId: ").append(layerId).append("\n");
sb.append(" sessionGuid: ").append(sessionGuid).append("\n");
sb.append(" creatorGuid: ").append(creatorGuid).append("\n");
sb.append(" creatorName: ").append(creatorName).append("\n");
sb.append(" creatorEmail: ").append(creatorEmail).append("\n");
sb.append(" box: ").append(box).append("\n");
sb.append(" pageNumber: ").append(pageNumber).append("\n");
sb.append(" annotationPosition: ").append(annotationPosition).append("\n");
sb.append(" range: ").append(range).append("\n");
sb.append(" svgPath: ").append(svgPath).append("\n");
sb.append(" type: ").append(type).append("\n");
sb.append(" access: ").append(access).append("\n");
sb.append(" replies: ").append(replies).append("\n");
sb.append(" createdOn: ").append(createdOn).append("\n");
sb.append(" fontColor: ").append(fontColor).append("\n");
sb.append(" penColor: ").append(penColor).append("\n");
sb.append(" penWidth: ").append(penWidth).append("\n");
sb.append(" penStyle: ").append(penStyle).append("\n");
sb.append(" backgroundColor: ").append(backgroundColor).append("\n");
sb.append(" fieldText: ").append(fieldText).append("\n");
sb.append(" fontFamily: ").append(fontFamily).append("\n");
sb.append(" fontSize: ").append(fontSize).append("\n");
sb.append("}\n");
return sb.toString();
}
}