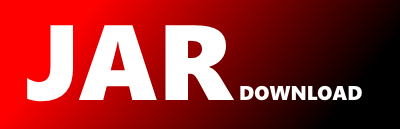
com.groupdocs.sdk.model.SubscriptionPlanInfo Maven / Gradle / Ivy
/**
* Copyright 2012 GroupDocs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.groupdocs.sdk.model;
import java.util.Date;
import com.groupdocs.sdk.model.BillingAddressInfo;
/**
*
*
* NOTE: This class is auto generated by the swagger code generator program. Do not edit the class manually.
*
*/
public class SubscriptionPlanInfo {
private Integer productId = null;
private String name = null;
private Integer userCount = null;
private String firstNameOnCard = null;
private String lastNameOnCard = null;
private String number = null;
private Date expirationDate = null;
private String cvv = null;
private BillingAddressInfo address = null;
private Double price = null;
private String currencyCode = null;
private Integer billingPeriod = null;
private String promoCode = null;
private String nextAssesmentDate = null;
public Integer getProductId() {
return productId;
}
public void setProductId(Integer productId) {
this.productId = productId;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getUserCount() {
return userCount;
}
public void setUserCount(Integer userCount) {
this.userCount = userCount;
}
public String getFirstNameOnCard() {
return firstNameOnCard;
}
public void setFirstNameOnCard(String firstNameOnCard) {
this.firstNameOnCard = firstNameOnCard;
}
public String getLastNameOnCard() {
return lastNameOnCard;
}
public void setLastNameOnCard(String lastNameOnCard) {
this.lastNameOnCard = lastNameOnCard;
}
public String getNumber() {
return number;
}
public void setNumber(String number) {
this.number = number;
}
public Date getExpirationDate() {
return expirationDate;
}
public void setExpirationDate(Date expirationDate) {
this.expirationDate = expirationDate;
}
public String getCvv() {
return cvv;
}
public void setCvv(String cvv) {
this.cvv = cvv;
}
public BillingAddressInfo getAddress() {
return address;
}
public void setAddress(BillingAddressInfo address) {
this.address = address;
}
public Double getPrice() {
return price;
}
public void setPrice(Double price) {
this.price = price;
}
public String getCurrencyCode() {
return currencyCode;
}
public void setCurrencyCode(String currencyCode) {
this.currencyCode = currencyCode;
}
public Integer getBillingPeriod() {
return billingPeriod;
}
public void setBillingPeriod(Integer billingPeriod) {
this.billingPeriod = billingPeriod;
}
public String getPromoCode() {
return promoCode;
}
public void setPromoCode(String promoCode) {
this.promoCode = promoCode;
}
public String getNextAssesmentDate() {
return nextAssesmentDate;
}
public void setNextAssesmentDate(String nextAssesmentDate) {
this.nextAssesmentDate = nextAssesmentDate;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SubscriptionPlanInfo {\n");
sb.append(" productId: ").append(productId).append("\n");
sb.append(" name: ").append(name).append("\n");
sb.append(" userCount: ").append(userCount).append("\n");
sb.append(" firstNameOnCard: ").append(firstNameOnCard).append("\n");
sb.append(" lastNameOnCard: ").append(lastNameOnCard).append("\n");
sb.append(" number: ").append(number).append("\n");
sb.append(" expirationDate: ").append(expirationDate).append("\n");
sb.append(" cvv: ").append(cvv).append("\n");
sb.append(" address: ").append(address).append("\n");
sb.append(" price: ").append(price).append("\n");
sb.append(" currencyCode: ").append(currencyCode).append("\n");
sb.append(" billingPeriod: ").append(billingPeriod).append("\n");
sb.append(" promoCode: ").append(promoCode).append("\n");
sb.append(" nextAssesmentDate: ").append(nextAssesmentDate).append("\n");
sb.append("}\n");
return sb.toString();
}
}