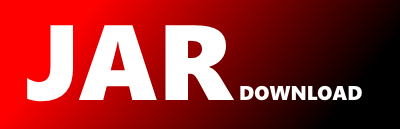
com.gruelbox.transactionoutbox.SimpleTransactionManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of transactionoutbox-core Show documentation
Show all versions of transactionoutbox-core Show documentation
A safe implementation of the transactional outbox pattern for Java (core library)
package com.gruelbox.transactionoutbox;
import com.gruelbox.transactionoutbox.spi.AbstractThreadLocalTransactionManager;
import com.gruelbox.transactionoutbox.spi.SimpleTransaction;
import com.gruelbox.transactionoutbox.spi.Utils;
import java.sql.Connection;
import java.sql.SQLException;
import lombok.experimental.SuperBuilder;
import lombok.extern.slf4j.Slf4j;
/**
* A simple {@link TransactionManager} implementation suitable for applications with no existing
* transaction management.
*/
@SuperBuilder
@Slf4j
final class SimpleTransactionManager
extends AbstractThreadLocalTransactionManager {
private final ConnectionProvider connectionProvider;
@Override
public T inTransactionReturnsThrows(
ThrowingTransactionalSupplier work) throws E {
return withTransaction(
atx -> {
T result = processAndCommitOrRollback(work, (SimpleTransaction) atx);
((SimpleTransaction) atx).processHooks();
return result;
});
}
private T processAndCommitOrRollback(
ThrowingTransactionalSupplier work, SimpleTransaction transaction) throws E {
try {
log.debug("Processing work");
T result = work.doWork(transaction);
transaction.flushBatches();
log.debug("Committing transaction");
transaction.commit();
return result;
} catch (Exception e) {
try {
log.warn(
"Exception in transactional block ({}{}). Rolling back. See later messages for detail",
e.getClass().getSimpleName(),
e.getMessage() == null ? "" : (" - " + e.getMessage()));
transaction.rollback();
} catch (Exception ex) {
log.warn("Failed to roll back", ex);
}
throw e;
}
}
private T withTransaction(ThrowingTransactionalSupplier work)
throws E {
try (Connection connection = connectionProvider.obtainConnection();
SimpleTransaction transaction = pushTransaction(new SimpleTransaction(connection, null))) {
log.debug("Got connection {}", connection);
boolean autoCommit = transaction.connection().getAutoCommit();
if (autoCommit) {
log.debug("Setting auto-commit false");
Utils.uncheck(() -> transaction.connection().setAutoCommit(false));
}
try {
return work.doWork(transaction);
} finally {
connection.setAutoCommit(autoCommit);
}
} catch (SQLException e) {
throw new RuntimeException(e);
} finally {
popTransaction();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy