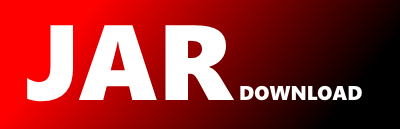
com.gruelbox.transactionoutbox.StubParameterContextTransactionManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of transactionoutbox-core Show documentation
Show all versions of transactionoutbox-core Show documentation
A safe implementation of the transactional outbox pattern for Java (core library)
package com.gruelbox.transactionoutbox;
import com.gruelbox.transactionoutbox.spi.ProxyFactory;
import com.gruelbox.transactionoutbox.spi.SimpleTransaction;
import com.gruelbox.transactionoutbox.spi.Utils;
import java.sql.Connection;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
import java.util.function.Supplier;
import lombok.extern.slf4j.Slf4j;
/**
* A stub transaction manager that assumes no underlying database, and a transaction context of the
* specified type.
*/
@Slf4j
public class StubParameterContextTransactionManager
implements ParameterContextTransactionManager {
private final Class contextClass;
private final Supplier contextFactory;
private final ConcurrentMap contextMap = new ConcurrentHashMap<>();
/**
* @param contextClass The class that represents the context. Must support equals/hashCode.
* @param contextFactory Generates context instances when transactions are started.
*/
public StubParameterContextTransactionManager(Class contextClass, Supplier contextFactory) {
this.contextClass = contextClass;
this.contextFactory = contextFactory;
}
@Override
public Transaction transactionFromContext(C context) {
return contextMap.get(context);
}
@Override
public final Class contextType() {
return contextClass;
}
@Override
public T inTransactionReturnsThrows(
ThrowingTransactionalSupplier work) throws E {
return withTransaction(
atx -> {
T result = work.doWork(atx);
((SimpleTransaction) atx).processHooks();
return result;
});
}
private T withTransaction(ThrowingTransactionalSupplier work)
throws E {
Connection mockConnection = Utils.createLoggingProxy(new ProxyFactory(), Connection.class);
C context = contextFactory.get();
try (SimpleTransaction transaction = new SimpleTransaction(mockConnection, context)) {
contextMap.put(context, transaction);
return work.doWork(transaction);
} finally {
contextMap.remove(context);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy