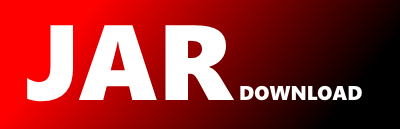
com.gruelbox.transactionoutbox.jooq.JooqTransactionManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of transactionoutbox-jooq Show documentation
Show all versions of transactionoutbox-jooq Show documentation
A safe implementation of the transactional outbox pattern for Java (jOOQ extension library)
package com.gruelbox.transactionoutbox.jooq;
import com.gruelbox.transactionoutbox.ParameterContextTransactionManager;
import com.gruelbox.transactionoutbox.ThreadLocalContextTransactionManager;
import com.gruelbox.transactionoutbox.TransactionManager;
import com.gruelbox.transactionoutbox.TransactionOutbox;
import org.jooq.Configuration;
import org.jooq.DSLContext;
/**
* Transaction manager which uses jOOQ's transaction management. In order to wire into JOOQ's
* transaction lifecycle, a slightly convoluted construction process is required which involves
* first creating a {@link JooqTransactionListener}, including it in the JOOQ {@link Configuration}
* while constructing the root {@link DSLContext}, and then finally linking the listener to the new
* {@link JooqTransactionManager}:
*
*
* DataSourceConnectionProvider connectionProvider = new DataSourceConnectionProvider(dataSource);
* DefaultConfiguration configuration = new DefaultConfiguration();
* configuration.setConnectionProvider(connectionProvider);
* configuration.setSQLDialect(SQLDialect.H2);
* configuration.setTransactionProvider(new ThreadLocalTransactionProvider(connectionProvider));
* JooqTransactionListener listener = JooqTransactionManager.createListener();
* configuration.set(listener);
* DSLContext dsl = DSL.using(configuration);
* return JooqTransactionManager.create(dsl, listener);
*/
public interface JooqTransactionManager extends TransactionManager {
/**
* Creates the {@link org.jooq.TransactionListener} to wire into the {@link DSLContext}. See
* class-level documentation for more detail.
*
* @return The transaction listener.
*/
static JooqTransactionListener createListener() {
return new JooqTransactionListener();
}
/**
* Creates a transaction manager which uses thread-local context. Attaches to the supplied {@link
* JooqTransactionListener} to receive notifications of transactions starting and finishing on the
* local thread so that {@link TransactionOutbox#schedule(Class)} can be called for methods that
* don't explicitly inject a {@link Configuration}, e.g.:
*
* dsl.transaction(() -> outbox.schedule(Foo.class).process("bar"));
*
* @param dslContext The DSL context.
* @param listener The listener, linked to the DSL context.
* @return The transaction manager.
*/
static ThreadLocalContextTransactionManager create(
DSLContext dslContext, JooqTransactionListener listener) {
var result = new ThreadLocalJooqTransactionManager(dslContext);
listener.setJooqTransactionManager(result);
return result;
}
/**
* Creates a transaction manager which uses explicitly-passed context, allowing multiple active
* contexts in the current thread and contexts which are passed between threads. Requires a {@link
* Configuration} for the transaction context or a {@link org.jooq.Transaction} to be used to be
* passed any method called via {@link TransactionOutbox#schedule(Class)}. Example:
*
*
* void doSchedule() {
* // ctx1 is used to write the request to the DB
* dsl.transaction(ctx1 -> outbox.schedule(getClass()).process("bar", ctx1));
* }
*
* // ctx2 is injected at run time
* void process(String arg, org.jooq.Configuration ctx2) {
* ...
* }
*
* Or:
*
*
* void doSchedule() {
* // tx1 is used to write the request to the DB
* transactionManager.inTransaction(tx1 -> outbox.schedule(getClass()).process("bar", tx1));
* }
*
* // tx2 is injected at run time
* void process(String arg, Transaction tx2) {
* ...
* }
*
* @param dslContext The DSL context.
* @return The transaction manager.
*/
static ParameterContextTransactionManager create(DSLContext dslContext) {
return new DefaultJooqTransactionManager(dslContext);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy