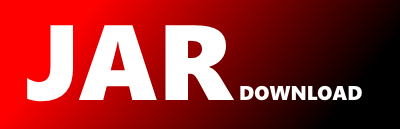
com.gtcgroup.justify.jpa.rule.JstConfigureJpaRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of justify-jpa Show documentation
Show all versions of justify-jpa Show documentation
JPA MODULE: PED Central is the home for the open-source �Justify� suite of software engineering modules. Justify seeks API alignment between Java 1.8+ application code and JUnit 5 test code.
/*
* [Licensed per the Open Source "MIT License".]
*
* Copyright (c) 2006 - 2017 by
* Global Technology Consulting Group, Inc. at
* http://gtcGroup.com
*
* Permission is hereby granted, free of charge, to any person obtaining
* a copy of this software and associated documentation files (the "Software"),
* to deal in the Software without restriction, including without limitation
* the rights to use, copy, modify, merge, publish, distribute, sublicense,
* and/or sell copies of the Software, and to permit persons to whom the
* Software is furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
* IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,
* DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
* TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE
* OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package com.gtcgroup.justify.jpa.rule;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import javax.persistence.EntityManager;
import javax.persistence.EntityManagerFactory;
import org.junit.Rule;
import org.junit.rules.TestRule;
import com.gtcgroup.justify.core.base.JstBaseRule;
import com.gtcgroup.justify.core.exception.internal.JustifyRuntimeException;
import com.gtcgroup.justify.core.exception.internal.RuleException;
import com.gtcgroup.justify.core.helper.internal.ReflectionUtilHelper;
import com.gtcgroup.justify.jpa.helper.JstBaseDataPopulator;
import com.gtcgroup.justify.jpa.helper.JstEntityManagerFactoryCacheHelper;
import com.gtcgroup.justify.jpa.po.JstTransactionJpaPO;
import com.gtcgroup.justify.jpa.rm.JstTransactionJpaRM;
/**
* This {@link Rule} class initializes persistence.
*
*
* Copyright (c) 2006 - 2017 by Global Technology Consulting Group, Inc. at
* gtcGroup.com .
*
*
* @author Marvin Toll
* @since v3.0
*/
public class JstConfigureJpaRule extends JstBaseRule {
public final static String KEY_DELIMITER = "_~_";
private static List DATA_POPULATOR_ALREADY_PROCESSED_LIST = new ArrayList();
private static List DATA_POPULATOR_TO_BE_PROCESSED_LIST = new ArrayList();
/**
* @return {@link TestRule}
*/
@SuppressWarnings("unchecked")
public static RULE withPersistenceUnit(final String persistenceUnitName) {
return (RULE)new JstConfigureJpaRule(persistenceUnitName);
}
/**
* @return {@link TestRule}
*/
@SuppressWarnings("unchecked")
public static RULE withPersistenceUnit(final String persistenceUnitName,
final Map persistencePropertyMap) {
return (RULE)new JstConfigureJpaRule(persistenceUnitName, persistencePropertyMap);
}
protected String persistenceUnitName;
protected Map persistencePropertyMapOrNull = null;
protected EntityManagerFactory entityManagerFactory;
protected boolean dataPopulatorSubmitted = false;
protected String persistenceKey;
/**
* Constructor - protected
*/
protected JstConfigureJpaRule(final String persistenceUnitName) {
this(persistenceUnitName, null);
}
/**
* Constructor - protected
*/
protected JstConfigureJpaRule(final String persistenceUnitName, final Map persistencePropertyMap) {
super();
this.persistenceKey =
JstEntityManagerFactoryCacheHelper.createEntityManagerFactory(persistenceUnitName, persistencePropertyMap);
this.persistenceUnitName = persistenceUnitName;
}
/**
* @see JstBaseRule#afterTM()
*/
@Override
public void afterTM() throws Throwable {
return;
}
/**
* @see JstBaseRule#beforeTM()
*/
@Override
public void beforeTM() {
if (0 != JstConfigureJpaRule.DATA_POPULATOR_TO_BE_PROCESSED_LIST.size()) {
for (final String listKey : JstConfigureJpaRule.DATA_POPULATOR_TO_BE_PROCESSED_LIST) {
final Class> dataPopulator = retrieveClassname(listKey);
processDataPopulator(dataPopulator, listKey);
JstConfigureJpaRule.DATA_POPULATOR_ALREADY_PROCESSED_LIST.add(listKey);
}
}
JstConfigureJpaRule.DATA_POPULATOR_TO_BE_PROCESSED_LIST.clear();
}
protected void processDataPopulator(final Class> populatorClass, final String listKey) {
EntityManager entityManager = null;
JstBaseDataPopulator dataPopulator;
try {
dataPopulator = (JstBaseDataPopulator)ReflectionUtilHelper
.instantiatePublicConstructorNoArgument(populatorClass);
} catch (final Exception e) {
JstConfigureJpaRule.DATA_POPULATOR_TO_BE_PROCESSED_LIST.remove(formatListKey(populatorClass));
throw (JustifyRuntimeException)e;
}
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy