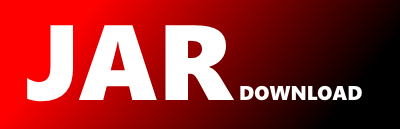
com.gu.zuora.soap.actions.Actions.scala Maven / Gradle / Ivy
package com.gu.zuora.soap.actions
import com.gu.i18n.Country
import com.gu.zuora.ZuoraSoapConfig
import com.gu.zuora.soap.DateTimeHelpers._
import com.gu.zuora.soap.models.Results._
import org.joda.time.LocalDate.now
import org.joda.time.{DateTime, LocalDate}
import scala.xml.{Elem, Null, _}
object Actions {
/*
* TODO: Split up these actions into simple models (In models/Commands) and XmlWriters
*/
case class Query(query: String, enableLog: Boolean = true) extends Action[QueryResult] {
override def additionalLogInfo = Map("Query" -> query)
val body =
{query}
override val enableLogging = enableLog
}
case class Login(apiConfig: ZuoraSoapConfig) extends Action[Authentication] {
override val authRequired = false
val body =
{apiConfig.username}
{apiConfig.password}
override def sanitized = "... "
}
/**
* See https://knowledgecenter.zuora.com/BC_Developers/SOAP_API/E_SOAP_API_Calls/update_call
*/
case class Update(zObjectId: String, objectType: String, fields: Seq[(String, String)]) extends Action[UpdateResult] {
val objectNamespace = s"ns2:$objectType"
override def additionalLogInfo = Map("ObjectType" -> objectType)
override protected val body: Elem =
{zObjectId}
{
fields.map { case (k, v) =>
Elem("ns2", k, Null, TopScope, false, Text(v))
}
}
}
case class CreateCreditCardReferencePaymentMethod(accountId: String, cardId: String, customerId: String, last4: String, cardCountry: Option[Country], expirationMonth: Int, expirationYear: Int, cardType: String) extends Action[CreateResult] {
override def additionalLogInfo = Map("AccountId" -> accountId)
val body =
{accountId}
{cardId}
{customerId}
CreditCardReferenceTransaction
{
cardCountry.map { country =>
{country.alpha2}
} getOrElse NodeSeq.Empty
}
{last4}
{expirationMonth}
{expirationYear}
{
// see CreditCardType allowed values
// https://knowledgecenter.zuora.com/DC_Developers/G_SOAP_API/E1_SOAP_API_Object_Reference/PaymentMethod
(cardType.toLowerCase.replaceAll(" ", "") match {
case "mastercard" => Some("MasterCard")
case "visa" => Some("Visa")
case "amex" => Some("AmericanExpress")
case "americanexpress" => Some("AmericanExpress")
case "discover" => Some("Discover")
case _ => None // TODO perhaps log invalid card type
}) map { cardType =>
{cardType}
} getOrElse NodeSeq.Empty
}
}
case class CreatePayPalReferencePaymentMethod(accountId: String, payPalBaid: String, email: String) extends Action[CreateResult] {
override def additionalLogInfo = Map("AccountId" -> accountId)
val body =
{accountId}
{payPalBaid}
{email}
ExpressCheckout
PayPal
}
sealed trait ZuoraNullableId
object Clear extends ZuoraNullableId
case class SetTo(value:String) extends ZuoraNullableId
case class UpdateAccountPayment(accountId: String, defaultPaymentMethodId: ZuoraNullableId, paymentGatewayName: String, autoPay: Option[Boolean], maybeInvoiceTemplateId: Option[String]) extends Action[UpdateResult] {
override def additionalLogInfo = Map("AccountId" -> accountId, "DefaultPaymentMethodId" -> defaultPaymentMethodId.toString, "PaymentGateway" -> paymentGatewayName, "AutoPay" -> autoPay.toString, "InvoiceTemplateOverride" -> maybeInvoiceTemplateId.mkString)
//We use two different vals here because order matters in the xml and zuora requires the clearing and settings lines to be in different places
val (setPaymentMethodLine, clearPaymentMethodLine) = defaultPaymentMethodId match {
case SetTo(p) => ({p} , NodeSeq.Empty)
case Clear => (NodeSeq.Empty, DefaultPaymentMethodId )
}
val autoPayLine = autoPay.map(ap => {ap} ).getOrElse(NodeSeq.Empty)
val invoiceTemplateLine = maybeInvoiceTemplateId.map(id => {id} ).getOrElse(NodeSeq.Empty)
val body =
{clearPaymentMethodLine}
{accountId}
{setPaymentMethodLine}
{invoiceTemplateLine}
{autoPayLine}
{paymentGatewayName}
}
/**
* A hack to get when a subscription charge dates will be effective. While it's possible to get this data from an
* Invoice of a subscription that charges the user immediately (e.g. annual partner sign up), it's not possible to get this for data
* for subscriptions that charge in the future (subs offer that charges 6 months in). To achieve the latter an amend
* call with preview can be used - this works for the first case too
*
* The dummy amend also sets the subscription to be evergreen - an infinite term length
* while the real subscriptions have one year terms. This is so we still get invoices for annual subs which
* have already been billed
*
*/
case class PreviewInvoicesViaAmend(numberOfPeriods: Int = 2)(subscriptionId: String, paymentDate: LocalDate = now) extends Action[AmendResult] {
override def additionalLogInfo = Map("SubscriptionId" -> subscriptionId, "PaymentDate" -> paymentDate.toString, "NumberOfPeriods" -> numberOfPeriods.toString)
val date = if(now isBefore paymentDate) paymentDate else now
val body = {
{date}
{date}
EVERGREEN
GetSubscriptionDetailsViaAmend
{subscriptionId}
TermsAndConditions
False
False
True
{numberOfPeriods}
}
}
case class PreviewInvoicesTillEndOfTermViaAmend(subscriptionId: String, paymentDate: LocalDate = now) extends Action[AmendResult] {
override def additionalLogInfo = Map("SubscriptionId" -> subscriptionId, "PaymentDate" -> paymentDate.toString)
val date = if(now isBefore paymentDate) paymentDate else now
val body = {
{date}
{date}
TERMED
GetSubscriptionDetailsViaAmend
{subscriptionId}
TermsAndConditions
False
False
True
True
}
}
case class CancelPlan(subscriptionId: String, subscriptionRatePlanId: String, date: LocalDate)
extends Action[AmendResult] {
override def additionalLogInfo = Map(
"SubscriptionId" -> subscriptionId,
"RatePlanId" -> subscriptionRatePlanId,
"Date" -> date.toString
)
val body = {
{date}
{date}
{date}
Cancellation
{subscriptionRatePlanId}
Completed
{subscriptionId}
Cancellation
}
}
case class DowngradePlan(subscriptionId: String, subscriptionRatePlanId: String, newRatePlanId: String, date: LocalDate) extends Action[AmendResult] {
override def additionalLogInfo = Map(
"SubscriptionId" -> subscriptionId,
"Existing RatePlanId" -> subscriptionRatePlanId,
"New RatePlanId" -> newRatePlanId,
"Date" -> date.toString
)
override val singleTransaction = true
val body = {
{date}
{date}
Downgrade
{subscriptionRatePlanId}
Completed
{subscriptionId}
RemoveProduct
{date}
{date}
Downgrade
{newRatePlanId}
Completed
{subscriptionId}
NewProduct
false
false
false
true
}
}
case class CreateFreeEventUsage(accountId: String, description: String, quantity: Int, subscriptionNumber: String) extends Action[CreateResult] {
val startDateTime = formatDateTime(DateTime.now)
override def additionalLogInfo = Map(
"AccountId" -> accountId,
"Description" -> description,
"Quantity" -> quantity.toString,
"SubscriptionNumber" -> subscriptionNumber
)
override protected val body: Elem =
{accountId}
{subscriptionNumber}
{quantity}
{startDateTime}
{description}
Events
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy