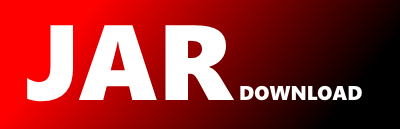
proguard.classfile.instruction.InstructionUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proguard-core Show documentation
Show all versions of proguard-core Show documentation
ProGuardCORE is a free library to read, analyze, modify, and write Java class files.
/*
* ProGuardCORE -- library to process Java bytecode.
*
* Copyright (c) 2002-2020 Guardsquare NV
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package proguard.classfile.instruction;
import proguard.classfile.TypeConstants;
/**
* Utility methods for converting between representations of names and descriptions.
*
* @author Eric Lafortune
*/
public class InstructionUtil {
/**
* Returns the internal type corresponding to the given 'newarray' type.
*
* @param arrayType Instruction.ARRAY_T_BOOLEAN
, Instruction.ARRAY_T_BYTE
*
, Instruction.ARRAY_T_CHAR
, Instruction.ARRAY_T_SHORT
,
* Instruction.ARRAY_T_INT
, Instruction.ARRAY_T_LONG
,
* Instruction.ARRAY_T_FLOAT
, or Instruction.ARRAY_T_DOUBLE
.
* @return TypeConstants.BOOLEAN
, TypeConstants.BYTE
,
* TypeConstants.CHAR
, TypeConstants.SHORT
, TypeConstants.INT
* , TypeConstants.LONG
, TypeConstants.FLOAT
, or
* TypeConstants.DOUBLE
.
*/
public static char internalTypeFromArrayType(byte arrayType) {
switch (arrayType) {
case Instruction.ARRAY_T_BOOLEAN:
return TypeConstants.BOOLEAN;
case Instruction.ARRAY_T_CHAR:
return TypeConstants.CHAR;
case Instruction.ARRAY_T_FLOAT:
return TypeConstants.FLOAT;
case Instruction.ARRAY_T_DOUBLE:
return TypeConstants.DOUBLE;
case Instruction.ARRAY_T_BYTE:
return TypeConstants.BYTE;
case Instruction.ARRAY_T_SHORT:
return TypeConstants.SHORT;
case Instruction.ARRAY_T_INT:
return TypeConstants.INT;
case Instruction.ARRAY_T_LONG:
return TypeConstants.LONG;
default:
throw new IllegalArgumentException("Unknown array type [" + arrayType + "]");
}
}
/**
* Returns the 'newarray' type constant for the given internal primitive type.
*
* @param internalType a primitive type TypeConstants.BOOLEAN
,
* TypeConstants.BYTE
, TypeConstants.CHAR
, TypeConstants.SHORT
*
, TypeConstants.INT
, TypeConstants.LONG
,
* TypeConstants.FLOAT
, or TypeConstants.DOUBLE
.
* @return the array type constant corresponding to the given primitive type;
* TypeConstants.BOOLEAN
, TypeConstants.BYTE
, TypeConstants.CHAR
*
, TypeConstants.SHORT
, TypeConstants.INT
,
* TypeConstants.LONG
, TypeConstants.FLOAT
, or TypeConstants.DOUBLE
*
.
* @see #internalTypeFromArrayType(byte)
*/
public static byte arrayTypeFromInternalType(char internalType) {
switch (internalType) {
case TypeConstants.BOOLEAN:
return Instruction.ARRAY_T_BOOLEAN;
case TypeConstants.BYTE:
return Instruction.ARRAY_T_BYTE;
case TypeConstants.CHAR:
return Instruction.ARRAY_T_CHAR;
case TypeConstants.SHORT:
return Instruction.ARRAY_T_SHORT;
case TypeConstants.INT:
return Instruction.ARRAY_T_INT;
case TypeConstants.LONG:
return Instruction.ARRAY_T_LONG;
case TypeConstants.FLOAT:
return Instruction.ARRAY_T_FLOAT;
case TypeConstants.DOUBLE:
return Instruction.ARRAY_T_DOUBLE;
default:
throw new IllegalArgumentException("Unknown primitive type [" + internalType + "]");
}
}
/**
* Check if an instruction opcode refers to a static call (i.e. no calling instance needed).
*
* @param opcode The instruction opcode to check
* @return False if this call requires a calling instance, true otherwise.
*/
public static boolean isStaticCall(byte opcode) {
switch (opcode) {
case Instruction.OP_INVOKESTATIC:
case Instruction.OP_INVOKEDYNAMIC:
return true;
default:
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy