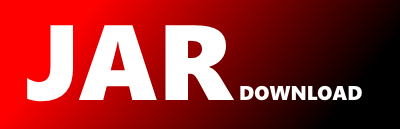
jakarta.ejb.TransactionAttributeType Maven / Gradle / Ivy
/*
* Copyright (c) 1997, 2020 Oracle and/or its affiliates. All rights reserved.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License v. 2.0, which is available at
* http://www.eclipse.org/legal/epl-2.0.
*
* This Source Code may also be made available under the following Secondary
* Licenses when the conditions for such availability set forth in the
* Eclipse Public License v. 2.0 are satisfied: GNU General Public License,
* version 2 with the GNU Classpath Exception, which is available at
* https://www.gnu.org/software/classpath/license.html.
*
* SPDX-License-Identifier: EPL-2.0 OR GPL-2.0 WITH Classpath-exception-2.0
*/
package jakarta.ejb;
/**
* The enum TransactionAttributeType
is used with the TransactionAttribute
annotation to
* specify whether the methods of a session bean or message driven bean are called with a valid transaction context.
*
*
* For a message-driven bean's message listener methods (or interface), only the REQUIRED
and
* NOT_SUPPORTED
values may be used.
*
*
* For an enterprise bean's timeout callback methods, only the REQUIRED
, REQUIRES_NEW
and
* NOT_SUPPORTED
values may be used.
*
*
* For a session bean's asynchronous business methods, only the REQUIRED
, REQUIRES_NEW
, and
* NOT_SUPPORTED
values may be used.
*
*
* For a singleton session bean's PostConstruct
and PreDestroy
lifecycle callback interceptor
* methods, only the REQUIRED
, REQUIRES_NEW
, and NOT_SUPPORTED
values may be
* used.
*
*
* If an enterprise bean implements the SessionSynchronization
interface or uses any of the session
* synchronization annotations, only the following values may be used for the transaction attributes of the bean's
* methods: REQUIRED
, REQUIRES_NEW
, MANDATORY
.
*
* @see TransactionAttribute
* @since EJB 3.0
*/
public enum TransactionAttributeType {
/**
* If a client invokes the enterprise bean's method while the client is associated with a transaction context, the
* container invokes the enterprise bean's method in the client's transaction context.
*
*
* If there is no existing transaction, an exception is thrown.
*/
MANDATORY,
/**
* If a client invokes the enterprise bean's method while the client is associated with a transaction context, the
* container invokes the enterprise bean's method in the client's transaction context.
*
*
* If the client invokes the enterprise bean's method while the client is not associated with a transaction context, the
* container automatically starts a new transaction before delegating a method call to the enterprise bean method.
*/
REQUIRED,
/**
* The container must invoke an enterprise bean method whose transaction attribute is set to REQUIRES_NEW
* with a new transaction context.
*
*
* If the client invokes the enterprise bean's method while the client is not associated with a transaction context, the
* container automatically starts a new transaction before delegating a method call to the enterprise bean business
* method.
*
*
* If a client calls with a transaction context, the container suspends the association of the transaction context with
* the current thread before starting the new transaction and invoking the method. The container resumes the suspended
* transaction association after the method and the new transaction have been completed.
*/
REQUIRES_NEW,
/**
* If the client calls with a transaction context, the container performs the same steps as described in the
* REQUIRED
case.
*
*
* If the client calls without a transaction context, the container performs the same steps as described in the
* NOT_SUPPORTED
case.
*
*
* The SUPPORTS
transaction attribute must be used with caution. This is because of the different
* transactional semantics provided by the two possible modes of execution. Only enterprise beans that will execute
* correctly in both modes should use the SUPPORTS
transaction attribute.
*/
SUPPORTS,
/**
* The container invokes an enterprise bean method whose transaction attribute NOT_SUPPORTED
with an
* unspecified transaction context.
*
*
* If a client calls with a transaction context, the container suspends the association of the transaction context with
* the current thread before invoking the enterprise bean's business method. The container resumes the suspended
* association when the business method has completed.
*/
NOT_SUPPORTED,
/**
* The client is required to call without a transaction context, otherwise an exception is thrown.
*/
NEVER
}