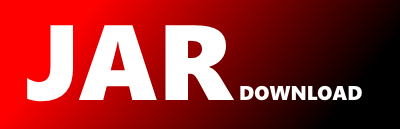
org.hibernate.collection.internal.PersistentArrayHolder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hibernate-core Show documentation
Show all versions of hibernate-core Show documentation
JPMS Module-Info's for a few of the Jakarta Libraries just until they add them in themselves
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later.
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.collection.internal;
import java.io.Serializable;
import java.lang.reflect.Array;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import org.hibernate.HibernateException;
import org.hibernate.engine.spi.SessionImplementor;
import org.hibernate.engine.spi.SharedSessionContractImplementor;
import org.hibernate.internal.CoreMessageLogger;
import org.hibernate.loader.CollectionAliases;
import org.hibernate.persister.collection.CollectionPersister;
import org.hibernate.type.Type;
import org.jboss.logging.Logger;
/**
* A persistent wrapper for an array. Lazy initialization
* is NOT supported. Use of Hibernate arrays is not really
* recommended.
*
* @author Gavin King
*/
public class PersistentArrayHolder extends AbstractPersistentCollection {
private static final CoreMessageLogger LOG = Logger.getMessageLogger(
CoreMessageLogger.class,
PersistentArrayHolder.class.getName()
);
protected Object array;
//just to help out during the load (ugly, i know)
private transient Class elementClass;
private transient java.util.List tempList;
/**
* Constructs a PersistentCollection instance for holding an array.
*
* @param session The session
* @param array The array (the persistent "collection").
*/
public PersistentArrayHolder(SharedSessionContractImplementor session, Object array) {
super( session );
this.array = array;
setInitialized();
}
/**
* Constructs a PersistentCollection instance for holding an array.
*
* @param session The session
* @param array The array (the persistent "collection").
*
* @deprecated {@link #PersistentArrayHolder(SharedSessionContractImplementor, Object)}
* should be used instead.
*/
@Deprecated
public PersistentArrayHolder(SessionImplementor session, Object array) {
this( (SharedSessionContractImplementor) session, array );
}
/**
* Constructs a PersistentCollection instance for holding an array.
*
* @param session The session
* @param persister The persister for the array
*/
public PersistentArrayHolder(SharedSessionContractImplementor session, CollectionPersister persister) {
super( session );
elementClass = persister.getElementClass();
}
/**
* Constructs a PersistentCollection instance for holding an array.
*
* @param session The session
* @param persister The persister for the array
*
* @deprecated {@link #PersistentArrayHolder(SharedSessionContractImplementor, CollectionPersister)}
* should be used instead.
*/
@Deprecated
public PersistentArrayHolder(SessionImplementor session, CollectionPersister persister) {
this( (SharedSessionContractImplementor) session, persister );
}
@Override
public Serializable getSnapshot(CollectionPersister persister) throws HibernateException {
// final int length = (array==null) ? tempList.size() : Array.getLength( array );
final int length = Array.getLength( array );
final Serializable result = (Serializable) Array.newInstance( persister.getElementClass(), length );
for ( int i=0; i deletes = new ArrayList<>();
final Serializable sn = getSnapshot();
final int snSize = Array.getLength( sn );
final int arraySize = Array.getLength( array );
int end;
if ( snSize > arraySize ) {
for ( int i=arraySize; i= Array.getLength( sn ) || Array.get( sn, i ) == null );
}
@Override
public boolean needsUpdating(Object entry, int i, Type elemType) throws HibernateException {
final Serializable sn = getSnapshot();
return i < Array.getLength( sn )
&& Array.get( sn, i ) != null
&& Array.get( array, i ) != null
&& elemType.isDirty( Array.get( array, i ), Array.get( sn, i ), getSession() );
}
@Override
public Object getIndex(Object entry, int i, CollectionPersister persister) {
return i;
}
@Override
public Object getElement(Object entry) {
return entry;
}
@Override
public Object getSnapshotElement(Object entry, int i) {
final Serializable sn = getSnapshot();
return Array.get( sn, i );
}
@Override
public boolean entryExists(Object entry, int i) {
return entry != null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy